C++11?е????????
???????????? ???????[ 2015/8/24 10:45:59 ] ??????????????????? .NET
??????????ú????????
????????????е?????????????????????????ú????????????????????????????????????????????????????????????????????????′???????main??????????????£?
void test_rvalue_rref(MyString &&str)
{
cout << "tmp object construct start" << endl;
MyString tmp = str;
cout << "tmp object construct finish" << endl;
}
int main() {
test_rvalue_rref(foo());
return 1;
}
// ??????
Constructor is called! this->_data: 28913680
Move Constructor is called! src: 28913680
DeConstructor is called!
tmp object construct start
Copy Constructor is called! src: 28913680 dst: 28913712
// ????????????????????????????????????????
tmp object construct finish
DeConstructor is called! this->_data: 28913712
DeConstructor is called! this->_data: 28913680
??????????????е?????????????????????????“Are you kidding me?????????????????????????????????????????”????????????ú????????????????
?????????????????????????????????????????????????????
??????????????????????????????????????????str???????????????????tmp???????????????????????????????????????tmp?????????????????????????????????str??????????????????????????????ú????и?????????????str??????????????????????????????????????????????????????????????????????????????tmp??????????治??????str??????????????std::move()??????str??????????????????????tmp???????????????????????????
???????????????????MyString b = foo()???????foo()?????????????????????????????????????b????????????????????
?????ù?????????????????????????????????ù????????????????д???????????????????
???????????
??????????????????????????????????????壬???????????????????????????????????????У??????????????л???????????????????????????????????????????????????У?????????????????????????????????????????????????????????????????????????????????????????????????????????????????????????????????????????????????????????????????????????const??????????????????????const????????????????const????????????????????????const???
??????????????????????????????????????
#include <iostream>
using namespace std;
void fun(int &) { cout << "lvalue ref" << endl; }
void fun(int &&) { cout << "rvalue ref" << endl; }
void fun(const int &) { cout << "const lvalue ref" << endl; }
void fun(const int &&) { cout << "const rvalue ref" << endl; }
template<typename T>
void PerfectForward(T t) { fun(t); }
int main()
{
PerfectForward(10);
// rvalue ref
int a;
PerfectForward(a);
// lvalue ref
PerfectForward(std::move(a));
// rvalue ref
const int b = 8;
PerfectForward(b);
// const lvalue ref
PerfectForward(std::move(b));
// const rvalue ref
return 0;
}
???????????????У???????????????PerfectForward??庯????????????????t??fun?????С??????????е?PerfectForward???????????????????????PerfectForward???????????????????????fun?????????void fun(int &)???????PerfectForward??????????β??????????????????????????????????????????????????????????????????????????????????????????????Ч???
????????????????PerfectForward??????????????????????????????????????ж?????????????????????????????PerfectForward????????????????????????????PerfectForward??????????????????????????????????????????????
??????????????const T &t??????????????????????????????const??????????????????κ?????????????????????????????const??????????????????const??????????????????const??????ú??const????????????????const??????
?????????????t???????const??????á?const????????????????????????
??????C++11???????????????????????????????????????????????????????????????????
??????????????????
????????????????????????????????????????????????
????typedef int& TR;
????int main()
????{
????int a = 1;
????int &b = a;
????int & &c = a;
????// ??????????????????????????????????
????TR &d = a;
????// ???typedef?????????????????????????
????return 1;
????}
??????C++?У?????????????ж??????????????????????????int & &c??????????????????????????????????????????????У??????????????????typedef??auto??????????????????????????????????????????????????????????????????????????????£????????????????ж?????????????????????????????????????á?????????????????????????????????????????????const????????й?????
????A& & => A&
????A& && => A&
????A&& & => A&
????A&& && => A&&
?????????????????????
??????????????????????????????????????????????庯??????庯???????????????????????????
????template<typename T>
????void foo(T&&);
?????????????????????????????????????????????????????????????????μ??????????????????????????????????????е????????????
???????foo????????????е?A???????????T???????A&???????????????????foo??????????A&??
???????foo????????????е?A???????????T???????A&&???????????????????foo??????????A&&??
??????????????????
?????????????????????????????????????????????????????????????????????????????д????????????????????
#include <iostream>
using namespace std;
void fun(int &) { cout << "lvalue ref" << endl; }
void fun(int &&) { cout << "rvalue ref" << endl; }
void fun(const int &) { cout << "const lvalue ref" << endl; }
void fun(const int &&) { cout << "const rvalue ref" << endl; }
//template<typename T>
//void PerfectForward(T t) { fun(t); }
// ??????????????????????е?????????????
template<typename T>
void PerfectForward(T &&t) { fun(static_cast<T &&>(t)); }
int main()
{
PerfectForward(10);
// rvalue ref???????t????????T &&
int a;
PerfectForward(a);
// lvalue ref???????t?????T &
PerfectForward(std::move(a));
// rvalue ref???????t?????T &&
const int b = 8;
PerfectForward(b);
// const lvalue ref???????t?????const T &
PerfectForward(std::move(b));
// const rvalue ref???????t?????const T &&
return 0;
}
??????????????????????????????????????????????????PerfectForward??庯???е?static_cast?????????static_cast?????????????????á????????μ???????????????????????????????const??????const?????
????// ??????????????????????????
????template<int && &&T>
????void PerfectForward(int && &&t) { fun(static_cast<int && &&>(t)); }
????// ?????????????ú?
????template<int &&T>
????void PerfectForward(int &&t) { fun(static_cast<int &&>(t)); }
????????????????з????????????е?????“????static_cast?????????????????????????”???????????????????????????ú?????????????????????????????????????????????????????????????????????????t?????????????????????????????????????fun?????д??????????????static_cast?????????????????
?????????C++11??????????????std::forward???????????????????????static_cast????????????????????std::forward??????汾??????
????template<typename T>
????void PerfectForward(T &&t) { fun(std::forward<T>(t)); }
??????????????std::move???????????????????????????????????std::forward????
???????????????????????漰???????????????????SPASVOС??(021-61079698-8054)?????????????????????????
??????
??C++????????????C++ lvalue??rvalueC++11????????C++???????????????C++?е?????????????????C++?????????C++???Windows????λ??C/C++???????????????????JAVA??C??C++??????????c++??python???????????????????????????????C++???????C++?е????????C++????????????????C++ ???????????????C++?????????????????????C++????????????
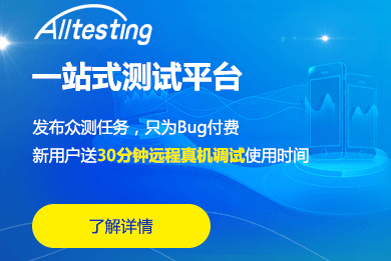
???·???
??????????????????
2023/3/23 14:23:39???д?ò??????????
2023/3/22 16:17:39????????????????????Щ??
2022/6/14 16:14:27??????????????????????????
2021/10/18 15:37:44???????????????
2021/9/17 15:19:29???·???????·
2021/9/14 15:42:25?????????????
2021/5/28 17:25:47??????APP??????????
2021/5/8 17:01:11????????
?????????App Bug???????????????????????Jmeter?????????QC??????APP????????????????app?????е????????jenkins+testng+ant+webdriver??????????????JMeter????HTTP???????Selenium 2.0 WebDriver ??????