C++11?е????????
???????????? ???????[ 2015/8/24 10:45:59 ] ??????????????????? .NET
????Constructor is called! this->_data: 29483024
????// middle??????????
????Copy Constructor is called! src: 29483024 dst: 29483056
????// ??????????????middle???????????????
????DeConstructor is called! this->_data: 29483024
????// middle?????????
????Copy Constructor is called! src: 29483056 dst: 29483024
????// a???????????????????????????
????DeConstructor is called! this->_data: 29483056
????// ???????????
????DeConstructor is called! this->_data: 29483024
????// a????????
???????????????У?????????????????????????????????Ч?????????C++???????????????????????????????????????????????в??????????_data?????????????????Ч???????????????????????????????????????????????C++11??????????????
??????C++11?н??????????·???????????????????????????????????????
????MyString(MyString &&str) {
????cout << "Move Constructor is called! src: " << (long)str._data << endl;
????_len = str._len;
????_data = str._data;
????str._data = nullptr;
????}
??????????????????????????str??????????????棬????????????????str?????????????nullptr?????????????????????????????????????????????????&&??????????????????????÷??????????????const?????????????????????????str???????
????????????????????????????????????????????????????????????????????????á??????????????????????????????????????????g++ test.cpp -o main -g -fno-elide-constructors –std=c++11??
????Constructor is called! this->_data: 22872080
????// middle??????
????Move Constructor is called! src: 22872080
????// ?????????????????????????middle???????????
????DeConstructor is called!
????// middle????????
????Move Constructor is called! src: 22872080
????// ????a????????????????????????????????
????DeConstructor is called!
????// ???????????
????DeConstructor is called! this->_data: 22872080
????// ????a????
???????????????????????????????н????????????棬??????÷??????????????
????C++98?е?????????
????????????????C++98?е?????????????????????????????????????????????????????????????????????????????????????????????????????????????????????????????????????&????????????????????????????????????????????????????????????????????????????????????????????????????????????????????????????????????????
// lvalues:
//
int i = 42;
i = 43;
// i?????
int* p = &i;
// i?????
int& foo();
foo() = 42;
// foo()?????????????????
int* p1 = &foo();
// foo()?????????????
// rvalues:
//
int foobar();
int j = 0;
j = foobar();
// foobar()?????
int* p2 = &foobar();
// ???????foobar()?????????????
j = 42;
// 42?????
????C++11??????ú????????
??????C++98????????????????const int &m = 1??????m?????????????????????????????????C++11?У?????????????????????&&?????????????????????ú????????????????????????????????????????????
void fun(MyString &str)
{
cout << "left reference" << endl;
}
void fun(MyString &&str)
{
cout << "right reference" << endl;
}
int main() {
MyString a("456");
fun(a);
// ????????????void fun(MyString &str)
fun(foo());
// ????????????void fun(MyString &&str)
return 1;
}
????????????????£??????????????ú????????????????????????????????????????????г?????????????????????ú???????????????????????????????????????????????????????????????????????????????????????塣?????????????????е?????????????楨????????????????
?????????????????????????????????????????????????????????????????????????£?????main???????????????????????????????????
MyString& operator=(MyString&& str) {
cout << "Move Operator= is called! src: " << (long)str._data << endl;
if (this != &str) {
if (_data != nullptr)
{
free(_data);
}
_len = str._len;
_data = str._data;
str._len = 0;
str._data = nullptr;
}
return *this;
}
int main() {
MyString b;
b = foo();
return 1;
}
// ???????????????????????????????
Constructor is called!
// ????b??????????
Constructor is called! this->_data: 14835728
// middle??????
Move Constructor is called! src: 14835728
// ?????????????????????middle??????
DeConstructor is called!
// middle????????
Move Operator= is called! src: 14835728
// ????b?????????????????????????
DeConstructor is called!
// ???????????
DeConstructor is called! this->_data: 14835728
// ????b????????????
??????C++?ж???????????????const?????Σ???const?????????????????????????????д??????????????????????????const??????á???const??????á?const??????ú??const??????????????????????????????????C++98??????μ??
??????const???????????????const????????????const???????const?????const????????????????const?????????????ж??
????const??????????????κ??????????const???????const?????const??????const?????????????????????а?const???????????????????????????е??????const int &a = 1????????????????????int &a = 1???
??????const??????ò???????κ??????const???????????const?????
????const????????????????????????????????? ??????????const MyString &&right_ref = foo()????????????????????????????????????壬?????????????????????????????????const????????????????????塣
??????????????????????????????????????????????????????????
????????????/???? ??const??? const??? ??const??? const??? ???
??????const??????? ?? ?? ?? ?? ??
????const??????? ?? ?? ?? ?? ?????????????const??????????????
??????const??????? ?? ?? ?? ?? C++11???????????????????????????????
????const????? ?? ?? ?? ?? ?????????壬????????????????
????????????????????????????foo?????????????????
?????????????void foo(MyString &str)??????????void fun(MyString &&str)??????????foo???????????????const?????
?????????????void foo(const MyString &str)??????????void fun(MyString &&str)??????????foo???????????????????????????????????const???????????????
?????????????void foo(MyString &&str)??????????void fun(MyString &str)????foo???????????????const?????
??????????????std::move()
?????????????????????????????????????????????????????????????????????????Ч????μ????????????????????н?????
??????C++98?е?swap???????????????£???ú????????????????????????е????a??b??c??????????????????????????塣
????template <class T>
????void swap ( T& a?? T& b )
????{
????T c(a);
????a=b;
????b=c;
????}
????????????ú?????????????????????????????????????????????????????????????????????????????????c?????????const???????????????a???const???????const??????ò???????κ??????
??????C++11??????????????std::move()????????????????ú????????????????????????????C++11?е?swap???????????????
????template <class T>
????void swap (T& a?? T& b)
????{
????T c(std::move(a));
????a=std::move(b);
????b=std::move(c);
????}
???????????move???????swap??????Ч??????????????????main?????????????:
int main() {
// move????
MyString d("123");
MyString e("456");
swap(d?? e);
return 1;
}
// ????????????????????????????????????
Constructor is called! this->_data: 38469648
// ????d????
Constructor is called! this->_data: 38469680
// ????e????
Move Constructor is called! src: 38469648
// swap?????е????c????????????????
Move Operator= is called! src: 38469680
// swap?????е????a??????????????????
Move Operator= is called! src: 38469648
// swap?????е????b??????????????????
DeConstructor is called!
// swap?????е????c????
DeConstructor is called! this->_data: 38469648
// ????e????
DeConstructor is called! this->_data: 38469680
// ????d????
???????????????????????漰???????????????????SPASVOС??(021-61079698-8054)?????????????????????????
??????
??C++????????????C++ lvalue??rvalueC++11????????C++???????????????C++?е?????????????????C++?????????C++???Windows????λ??C/C++???????????????????JAVA??C??C++??????????c++??python???????????????????????????????C++???????C++?е????????C++????????????????C++ ???????????????C++?????????????????????C++????????????
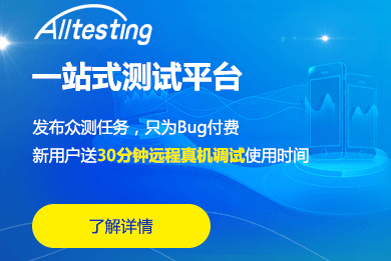
???·???
??????????????????
2023/3/23 14:23:39???д?ò??????????
2023/3/22 16:17:39????????????????????Щ??
2022/6/14 16:14:27??????????????????????????
2021/10/18 15:37:44???????????????
2021/9/17 15:19:29???·???????·
2021/9/14 15:42:25?????????????
2021/5/28 17:25:47??????APP??????????
2021/5/8 17:01:11????????
?????????App Bug???????????????????????Jmeter?????????QC??????APP????????????????app?????е????????jenkins+testng+ant+webdriver??????????????JMeter????HTTP???????Selenium 2.0 WebDriver ??????