C#???????????????????
???????????? ???????[ 2015/5/12 11:54:16 ] ???????????????
?????????????
using System;
using System.Collections.Generic;
using System.Text;
using System.Runtime.InteropServices;
using System.Security.Principal;
using System.IO;
namespace Test
{
public class Test
{
// logon types
const int LOGON32_LOGON_INTERACTIVE = 2;
const int LOGON32_LOGON_NETWORK = 3;
const int LOGON32_LOGON_NEW_CREDENTIALS = 9;
// logon providers
const int LOGON32_PROVIDER_DEFAULT = 0;
const int LOGON32_PROVIDER_WINNT50 = 3;
const int LOGON32_PROVIDER_WINNT40 = 2;
const int LOGON32_PROVIDER_WINNT35 = 1;
[DllImport("advapi32.dll"?? CharSet = CharSet.Auto?? SetLastError = true)]
public static extern int LogonUser(String lpszUserName??
String lpszDomain??
String lpszPassword??
int dwLogonType??
int dwLogonProvider??
ref IntPtr phToken);
[DllImport("advapi32.dll"?? CharSet = CharSet.Auto?? SetLastError = true)]
public static extern int DuplicateToken(IntPtr hToken??
int impersonationLevel??
ref IntPtr hNewToken);
[DllImport("advapi32.dll"?? CharSet = CharSet.Auto?? SetLastError = true)]
public static extern bool RevertToSelf();
[DllImport("kernel32.dll"?? CharSet = CharSet.Auto)]
public static extern bool CloseHandle(IntPtr handle);
private WindowsImpersonationContext impersonationContext;
public bool impersonateValidUser(String userName?? String domain?? String password)
{
WindowsIdentity tempWindowsIdentity;
IntPtr token = IntPtr.Zero;
IntPtr tokenDuplicate = IntPtr.Zero;
if (RevertToSelf())
{
// ???????LOGON32_LOGON_NEW_CREDENTIALS??????????????
// ??????????????????????????????????????????????????
// ????LOGON32_LOGON_INTERACTIVE
if (LogonUser(userName?? domain?? password?? LOGON32_LOGON_NEW_CREDENTIALS??
LOGON32_PROVIDER_DEFAULT?? ref token) != 0)
{
if (DuplicateToken(token?? 2?? ref tokenDuplicate) != 0)
{
tempWindowsIdentity = new WindowsIdentity(tokenDuplicate);
impersonationContext = tempWindowsIdentity.Impersonate();
if (impersonationContext != null)
{
System.AppDomain.CurrentDomain.SetPrincipalPolicy(PrincipalPolicy.WindowsPrincipal);
IPrincipal pr = System.Threading.Thread.CurrentPrincipal;
IIdentity id = pr.Identity;
CloseHandle(token);
CloseHandle(tokenDuplicate);
return true;
}
}
}
}
if (token != IntPtr.Zero)
CloseHandle(token);
if (tokenDuplicate != IntPtr.Zero)
CloseHandle(tokenDuplicate);
return false;
}
public void undoImpersonation()
{
impersonationContext.Undo();
}
public void TestFunc()
{
bool isImpersonated = false;
try
{
if (impersonateValidUser("UserName"?? "Domain"?? "Password"))
{
isImpersonated = true;
//do what you want now?? as the special user
// ...
File.Copy(@"\192.168.1.48generals ow.htm"?? "c:\now.htm"?? true);
}
}
finally
{
if (isImpersonated)
undoImpersonation();
}
}
}
}
?????塢???
????????????????Shell????Net Use?????е????
??????????????????Щ???????????????????????????
????????????????г???????????????????????????С???????????
??????????????????????????????????Щ????????????????????????е??????????????????
???????????????
?????????????????google??????????Щ??????????????????????????????????С????
??????Щ?????????????????棺
????1?????????????????????????????????????
????????
????public static extern int LogonUser(String lpszUserName??
????String lpszDomain??
????String lpszPassword??
????int dwLogonType??
????int dwLogonProvider??
????ref IntPtr phToken);
?????е?dwLogonType????????????????LOGON32_LOGON_NEW_CREDENTIALS??
????????????????LOGON32_LOGON_INTERACTIVE??
????2????????????????????
????a??????
????public static extern int LogonUser(String lpszUserName??
????String lpszDomain??
????String lpszPassword??
????int dwLogonType??
????int dwLogonProvider??
????ref IntPtr phToken);
?????е?lpszUserName??lpszDomain??lpszPassword?д?????
????????????????????????β????????????????????????????????????????????÷????
????LogonUser("myname"?? "192.168.1.48"?? "password"?? LOGON32_LOGON_NEW_CREDENTIALS??
????LOGON32_PROVIDER_DEFAULT?? ref token);
????????β??????????????????MyDomain?е?????????????????????????????????????
????LogonUser("myname"?? "MyDomain"?? "password"?? LOGON32_LOGON_NEW_CREDENTIALS??
????LOGON32_PROVIDER_DEFAULT?? ref token);
???????????????MyDomain??????IP?????
????b?????磺
?????ο????????
????string remote = @"\192.168.1.48generals";
????string local = @"P:";
????string username = @"DomainUserName";
????string password = @"Password";
???????@"\192.168.1.48generals"???@"\192.168.1.48generals”??????
????????????е???????????@"DomainUserName"???@"UserName"??????
???????????????????????漰???????????????????SPASVOС??(021-61079698-8054)?????????????????????????
??????
???????C#?е?StringC# Socket???????????????????C#?????????????????????????C#???????C#????????????Log4net??????δ????C#?????????????C#?????????????????C#???????????????C#????????????????C#7 ?е?Tuple??????C#??TypeScript - GeneratorC# ?????????細(xì)??????????C#?е???н???C#??MySQL?????????μ??Ч?????д??C#????C++??dll???C#??VS2010????е??????
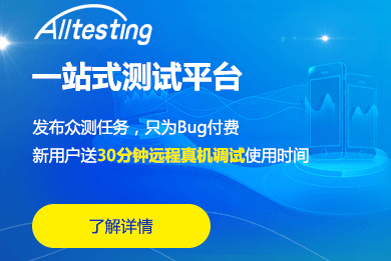
???·???
??????????????????
2023/3/23 14:23:39???д?ò??????????
2023/3/22 16:17:39????????????????????Щ??
2022/6/14 16:14:27??????????????????????????
2021/10/18 15:37:44???????????????
2021/9/17 15:19:29???·???????·
2021/9/14 15:42:25?????????????
2021/5/28 17:25:47??????APP??????????
2021/5/8 17:01:11????????
?????????App Bug???????????????????????Jmeter?????????QC??????APP????????????????app?????е????????jenkins+testng+ant+webdriver??????????????JMeter????HTTP???????Selenium 2.0 WebDriver ??????