C#???????????????????
???????????? ???????[ 2015/5/12 11:54:16 ] ???????????????
????????????WNetAddConnection2??WNetAddConnection3????NetUseAdd?????????д??????
using System;
using System.Collections.Generic;
using System.Text;
using System.Runtime.InteropServices;
namespace WindowsApplication1
{
public class MyMap
{
[DllImport("mpr.dll"?? EntryPoint = "WNetAddConnection2")]
public static extern uint WNetAddConnection2(
[In] NETRESOURCE lpNetResource??
string lpPassword??
string lpUsername??
uint dwFlags);
[DllImport("Mpr.dll")]
public static extern uint WNetCancelConnection2(
string lpName??
uint dwFlags??
bool fForce);
[StructLayout(LayoutKind.Sequential)]
public class NETRESOURCE
{
public int dwScope;
public int dwType;
public int dwDisplayType;
public int dwUsage;
public string LocalName;
public string RemoteName;
public string Comment;
public string Provider;
}
// remoteNetworkPath format: @"\192.168.1.48sharefolder"
// localDriveName format: @"E:"
public static bool CreateMap(string userName?? string password?? string remoteNetworkPath?? string localDriveName)
{
NETRESOURCE myNetResource = new NETRESOURCE();
myNetResource.dwScope = 2; //2:RESOURCE_GLOBALNET
myNetResource.dwType = 1; //1:RESOURCETYPE_ANY
myNetResource.dwDisplayType = 3; //3:RESOURCEDISPLAYTYPE_GENERIC
myNetResource.dwUsage = 1; //1: RESOURCEUSAGE_CONNECTABLE
myNetResource.LocalName = localDriveName;
myNetResource.RemoteName = remoteNetworkPath;
myNetResource.Provider = null;
uint nret = WNetAddConnection2(myNetResource?? password?? userName?? 0);
if (nret == 0)
return true;
else
return false;
}
// localDriveName format: @"E:"
public static bool DeleteMap(string localDriveName)
{
uint nret = WNetCancelConnection2(localDriveName?? 1?? true);
if (nret == 0)
return true;
else
return false;
}
public void test()
{
// ???
// remote??local??username??????????????????????????
string remote = @"\192.168.1.48generals";
string local = @"P:";
string username = @"DomainUserName";
string password = @"Password";
bool ret = MyMap.CreateMap(username?? password?? remote?? local);
if (ret)
{
//do what you want:
// ...
//File.Copy("q:\test.htm"?? "c:\test.htm");
MyMap.DeleteMap(local);
}
}
}
}
???????????WebClient??
????????WebClient??????????????????????????http:??https:??file:?????URI???????????WebClient?????????????
???????System.Net??????????????′????????????
private void Test1()
{
try
{
WebClient client = new WebClient();
NetworkCredential cred = new NetworkCredential("username"?? "password"?? "172.16.0.222");
client.Credentials = cred;
client.DownloadFile("file://172.16.0.222/test/111.txt"?? "111.txt");
}
catch (Exception ex)
{
// ?????????????????????????????г??????Time out????
}
}
public void Test2()
{
try
{
WebClient client = new WebClient();
NetworkCredential cred = new NetworkCredential("username"?? "password"?? "domain");
client.Credentials = cred;
client.DownloadFile("file://172.16.0.222/test/111.txt"?? "111.txt");
}
catch (Exception ex)
{
// ?????????????????????????????г??????Time out????
}
}
??????????????WebRequest??FileWebRequest???
WebRequest req = WebRequest.Create("file://138.12.12.14/generals/test.htm");
NetworkCredential cred = new NetworkCredential("username"?? "password"?? "IP");
req.Credentials = cred;
WebResponse response = req.GetResponse();
Stream strm = response.GetResponseStream();
StreamReader r = new StreamReader(strm);
... ...
???????????????????????漰???????????????????SPASVOС??(021-61079698-8054)?????????????????????????
??????
???????C#?е?StringC# Socket???????????????????C#?????????????????????????C#???????C#????????????Log4net??????δ????C#?????????????C#?????????????????C#???????????????C#????????????????C#7 ?е?Tuple??????C#??TypeScript - GeneratorC# ?????????細??????????C#?е???н???C#??MySQL?????????μ??Ч?????д??C#????C++??dll???C#??VS2010????е??????
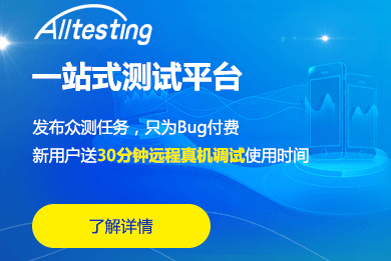
???·???
??????????????????
2023/3/23 14:23:39???д?ò??????????
2023/3/22 16:17:39????????????????????Щ??
2022/6/14 16:14:27??????????????????????????
2021/10/18 15:37:44???????????????
2021/9/17 15:19:29???·???????·
2021/9/14 15:42:25?????????????
2021/5/28 17:25:47??????APP??????????
2021/5/8 17:01:11????????
?????????App Bug???????????????????????Jmeter?????????QC??????APP????????????????app?????е????????jenkins+testng+ant+webdriver??????????????JMeter????HTTP???????Selenium 2.0 WebDriver ??????