C++?е?????????????
???????????? ???????[ 2014/5/7 9:26:25 ] ????????C++ ??? ???
?????????????????????????????е??С???????????????е?????????????麯?????????磬
1 void DoSomething(Widget& w)
2 {
3 if( w.size() > 0 && w != someNastyWidget)
4 {
5 Widget temp(w);
6 temp.normalize();
7 temp.swap(w);
8 }
9 }
?????????????????????
????????w????????????Widget??????w???????Widget?????????????????????????Щ????????Widget.h?????????Щ?????????????
????Widget??????????????????????????Widget?????п??????麯?????????????normalize???????????????????????????????
???????????????
??????????????????????????????????????????????????壬????????л???????????????κι????????????????????????????????????????????????????????????????????Щ???????????????壬????????????????????????????????????????μ?????????????????????
???????????????????У?????????????????????麯?????????????????????????????У????????????????????????????????
??????????
1 namespace StaticPoly
2 {
3 class Line
4 {
5 public:
6 void Draw()const{ std::cout << "Line Draw()
"; }
7 };
8
9 class Circle
10 {
11 public:
12 void Draw(const char* name=NULL)const{ std::cout << "Circle Draw()
"; }
13 };
14
15 class Rectangle
16 {
17 public:
18 void Draw(int i = 0)const{ std::cout << "Rectangle Draw()
"; }
19 };
20
21 template<typename Geometry>
22 void DrawGeometry(const Geometry& geo)
23 {
24 geo.Draw();
25 }
26
27 template<typename Geometry>
28 void DrawGeometry(std::vector<Geometry> vecGeo)
29 {
30 const size_t size = vecGeo.size();
31 for(size_t i = 0; i < size; ++i)
32 vecGeo[i].Draw();
33 }
34 }
35
36 void test_static_polymorphism()
37 {
38 StaticPoly::Line line;
39 StaticPoly::Circle circle;
40 StaticPoly::Rectangle rect;
41 StaticPoly::DrawGeometry(circle);
42
43 std::vector<StaticPoly::Line> vecLines;
44 StaticPoly::Line line2;
45 StaticPoly::Line line3;
46 vecLines.push_back(line);
47 vecLines.push_back(line2);
48 vecLines.push_back(line3);
49 //vecLines.push_back(&circle); //??????????????????????????
50 //vecLines.push_back(&rect); //??????????????????????????
51 StaticPoly::DrawGeometry(vecLines);
52
53 std::vector<StaticPoly::Circle> vecCircles;
54 vecCircles.push_back(circle);
55 StaticPoly::DrawGeometry(circle);
56 }
???????????????????????????????????е?????????????????????????????????????????????????????????????????????????????????????????Ч???????? ???磬
1 template<typename Widget??typename Other>
2 void DoSomething(Widget& w?? const Other& someNasty)
3 {
4 if( w.size() > 0 && w != someNasty) //someNastyT????????T????????????????????
5 {
6 Widget temp(w);
7 temp.normalize();
8 temp.swap(w);
9 }
10 }
???????????
????????T??????size??normalize??swap??????copy??????????????в?????
????????T??????????????о??????????????ò?????????????????????????????????????
?????????
????size??????????????????????????10???????????????????????????????????????????????????X???????X?????int????????10?????????????????operator >?????operator>????????????X???????????????????????????????X??????Y?????????????Y????????int?????????(?????????????????????????????????????)??
???????????T??????????operator!=????????T??????X???????someNastyT??????Y?????????X??Y??????в???????ɡ?
?????????????????????
??????????
???????
?????????????????????????????Ч????????????????????????
?????к??????????????????????????????????????????????????????
??????????????????????????C++????????????????????????STL??
???????
?????????????????????????????????????????????????????????????????????????????????????????????
??????????????????????
??????????
???????
????OO???????????????????????
???????????????????
???????????????????????????????????
???????
???????????????????????????????????
????????????????麯?????????
????????????????????????????????????Σ?
?????????
??????????????????????????????????????????????????????????????????С??麯??????
???????????н???????????????????????????????麯?????????????????????н??????????????Ч????????????????????????????????
?????????
???????????????????????/????????????????/??????????
?????????????????????????????嶨?????????????????????????????麯????????????????????
??????????β???????д???
????Static_Dynamic_Polymorphism.cpp
??????
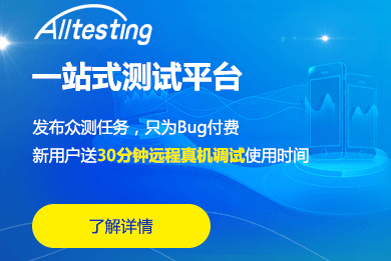
???·???
??????????????????
2023/3/23 14:23:39???д?ò??????????
2023/3/22 16:17:39????????????????????Щ??
2022/6/14 16:14:27??????????????????????????
2021/10/18 15:37:44???????????????
2021/9/17 15:19:29???·???????·
2021/9/14 15:42:25?????????????
2021/5/28 17:25:47??????APP??????????
2021/5/8 17:01:11