C++?е?????????????
???????????? ???????[ 2014/5/7 9:26:25 ] ????????C++ ??? ???
??????C++???????????????????(multiparadigm programming lauguage)????????????????(procedural)????????????(object-oriented)?????????(functional)?????????(generic)?????????(metaprogramming)??????? ??Щ??????????C++???????????е???????????????????????Щ???????????????????????У???????????????????????μ????????????????????????????????? C++?????????????????????????????????麯??????塢??????????????????????????????????????????????????????????????
??????????????????е????????????????????????????????????????????????????????????????????????????????????????????????????????????????????????????????????????????????滻????C++????????????????????????“????”??
???????????????
???????????????????????????????????????????????????????????????????У?????Щ???????????????????????麯????????????????д??Щ?麯??????????????????????????????????????????????????????????????Щ???????麯??????????????????????????????????
?????????????????????????????????麯??????????????????????????????????????????????????????????????????????????????????????????????????????????????
??????????
1 namespace DynamicPoly
2 {
3 class Geometry
4 {
5 public:
6 virtual void Draw()const = 0;
7 };
8
9 class Line : public Geometry
10 {
11 public:
12 virtual void Draw()const{ std::cout << "Line Draw()
"; }
13 };
14
15 class Circle : public Geometry
16 {
17 public:
18 virtual void Draw()const{ std::cout << "Circle Draw()
"; }
19 };
20
21 class Rectangle : public Geometry
22 {
23 public:
24 virtual void Draw()const{ std::cout << "Rectangle Draw()
"; }
25 };
26
27 void DrawGeometry(const Geometry *geo)
28 {
29 geo->Draw();
30 }
31
32 //????????????????????????????????????
33 void DrawGeometry(std::vector<DynamicPoly::Geometry*> vecGeo)
34 {
35 const size_t size = vecGeo.size();
36 for(size_t i = 0; i < size; ++i)
37 vecGeo[i]->Draw();
38 }
39 }
40
41 void test_dynamic_polymorphism()
42 {
43 DynamicPoly::Line line;
44 DynamicPoly::Circle circle;
45 DynamicPoly::Rectangle rect;
46 DynamicPoly::DrawGeometry(&circle);
47
48 std::vector<DynamicPoly::Geometry*> vec;
49 vec.push_back(&line);
50 vec.push_back(&circle);
51 vec.push_back(&rect);
52 DynamicPoly::DrawGeometry(vec);
53 }
??????
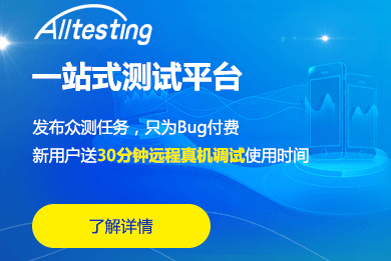
???·???
??????????????????
2023/3/23 14:23:39???д?ò??????????
2023/3/22 16:17:39????????????????????Щ??
2022/6/14 16:14:27??????????????????????????
2021/10/18 15:37:44???????????????
2021/9/17 15:19:29???·???????·
2021/9/14 15:42:25?????????????
2021/5/28 17:25:47??????APP??????????
2021/5/8 17:01:11