??LuaBridge?Lua??C/C++????
???????????? ???????[ 2014/4/2 9:37:22 ] ????????C++ Lua ???
?????????????????????Lua?????test?? test.detail????test.utility????????????endNamespace????????????????????????table???????????????????namespace????????namespace?????ɡ? All LuaBridge functions which create registrations return an object upon which subsequent registrations can be made??allowing for an unlimited number of registrations to be chained together using the dot operator???????namespace?У????????????????????LuaBridge???????ж????????????namespace????????????????????????????????δ?????????
<span style="font-size:14px;"> getGlobalNamespace (L)
.beginNamespace ("test")
.addFunction ("foo"?? foo)
.endNamespace ();
getGlobalNamespace (L)
.beginNamespace ("test")
.addFunction ("bar"?? bar)
.endNamespace ();</span>
??????
<span style="font-size:14px;"> getGlobalNamespace (L)
.beginNamespace ("test")
.addFunction ("foo"?? foo)
.addFunction ("bar"?? bar)
.endNamespace ();</span>
Data?? Properties?? Functions?? and CFunctions
????Data?? Properties?? Functions?? and CFunctions???????????addVariable???? addProperty?? addFunction?? and addCFunction???????Lua????е?????????????LuaBridge???????????????????????????????????顣?????????????????????????????LuaBridge??????8????????Pointers?? references?? and objectsof class type as parameters are treated specially???????????C++??????????壺
<span style="font-size:14px;"> int globalVar;
static float staticVar;
std::string stringProperty;
std::string getString () { return stringProperty; }
void setString (std::string s) { stringProperty = s; }
int foo () { return 42; }
void bar (char const*) { }
int cFunc (lua_State* L) { return 0; }</span>
?????????Lua?????Щ??????????????????????·??????????
<span style="font-size:14px;"> getGlobalNamespace (L)
.beginNamespace ("test")
.addVariable ("var1"?? &globalVar)
.addVariable ("var2"?? &staticVar?? false) // read-only
.addProperty ("prop1"?? getString?? setString)
.addProperty ("prop2"?? getString) // read only
.addFunction ("foo"?? foo)
.addFunction ("bar"?? bar)
.addCFunction ("cfunc"?? cFunc)
.endNamespace ();
</span>
????Variables????????????????????????????false?????Variables??????Lua???????????????????true??Properties???????????????set????????????????read-only??
??????????????????????????Lua????Ч???
????<span style="font-size:14px;"> test -- a namespace??????????table?????涼??table?е???
test.var1 -- a lua_Number variable
test.var2 -- a read-only lua_Number variable
test.prop1 -- a lua_String property
test.prop2 -- a read-only lua_String property
test.foo -- a function returning a lua_Number
test.bar -- a function taking a lua_String as a parameter
test.cfunc -- a function with a variable argument list and multi-return</span>
???????test.prop1??test.prop2?????C++???????????????test.prop2??read-only??????????ж?test.prop2??????????????????run-time error??????Lua?????·??????
<span style="font-size:14px;"> test.var1 = 5 -- okay
test.var2 = 6 -- error: var2 is not writable
test.prop1 = "Hello" -- okay
test.prop1 = 68 -- okay?? Lua converts the number to a string.
test.prop2 = "bar" -- error: prop2 is not writable
test.foo () -- calls foo and discards the return value
test.var1 = foo () -- calls foo and stores the result in var1
test.bar ("Employee") -- calls bar with a string
test.bar (test) -- error: bar expects a string not a table</span>
Class Objects
??????????????beginClass??deriveClass???????endClass??????????????????????????beginClass????????????????????deriveClass?????????Ρ??????????deriveClass?????????????????????????beginClass??
<span style="font-size:14px;"> class A {
public:
A() { printf("A constructor
");}
static int staticData;
static int getStaticData() {return staticData;}
static float staticProperty;
static float getStaticProperty () { return staticProperty; }
static void setStaticProperty (float f) { staticProperty = f; }
static int staticCFunc (lua_State *L) { return 0; }
std::string dataMember;
char dataProperty;
char getProperty () const { return dataProperty; }
void setProperty (char v) { dataProperty = v; }
void func1 () {printf("func1 In Class A
"); }
virtual void virtualFunc () {printf("virtualFunc In Class A
"); }
int cfunc (lua_State* L) { printf("cfunc In Class A
"); return 0; }
};
class B : public A {
public:
B() { printf("B constructor
");}
double dataMember2;
void func1 () {printf("func1 In Class B
"); }
void func2 () { printf("func2 In Class B
"); }
void virtualFunc () {printf("virtualFunc In Class B
"); }
};
int A::staticData = 3;
float A::staticProperty = 0.5;</span>
??????
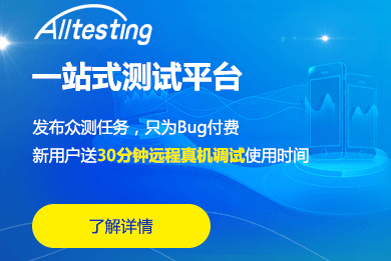
???·???
??????????????????
2023/3/23 14:23:39???д?ò??????????
2023/3/22 16:17:39????????????????????Щ??
2022/6/14 16:14:27??????????????????????????
2021/10/18 15:37:44???????????????
2021/9/17 15:19:29???·???????·
2021/9/14 15:42:25?????????????
2021/5/28 17:25:47??????APP??????????
2021/5/8 17:01:11