Repository?????????
???????????? ???????[ 2016/4/6 10:17:35 ] ?????????????????? ???????
??????2????DbSettings?а??趨??????????????????????????DbSettings???
1 namespace Example.Infrastructure.Repository
2 {
3 public class DbSettings
4 {
5 public DbSettings()
6 {
7 this.RowVersionNname = "Version";
8 }
9
10 public string NameOrConnectionString { get; set; }
11
12 public string RowVersionNname { get; set; }
13 public bool Debug { get; set; }
14
15 public bool UnitTest { get; set; }
16
17 public IDbConnectionFactory DbConnectionFactory { get; set; }
18
19 public List<object> EntityMaps { get; set; } = new List<object>();
20
21 public List<object> ComplexMaps { get; set; } = new List<object>();
22 }
23 }
????3.????SqlServerDbContext??VersionDbContext???????????????????????MySql?????????????????RowVersion??????
????(1)??????SqlServer??SqlServeCe??SqlServerDbContext
1 namespace Example.Infrastructure.Repository
2 {
3 public class SqlServerDbContext : EfDbContext
4 {
5 private DbSettings _dbSettings;
6
7 public SqlServerDbContext(IConfiguration configuration?? ILogger logger?? DbSettings dbSettings)
8 : base(configuration?? logger?? dbSettings)
9 {
10 this._dbSettings = dbSettings;
11 }
12
13 protected override void OnModelCreating(DbModelBuilder modelBuilder)
14 {
15 base.OnModelCreating(modelBuilder);
16 modelBuilder.Properties().Where(o => o.Name == this._dbSettings.RowVersionNname).Configure(o => o.IsRowVersion());
17 base.SetInitializer<SqlServerDbContext>();
18 }
19 }
20 }
????(2)??????Myql??Sqlite????????VersionDbContext????????????Version?????GUID????汾???
1 namespace Example.Infrastructure.Repository
2 {
3 public class VersionDbContext : EfDbContext
4 {
5 private DbSettings _dbSettings;
6
7 public VersionDbContext(IConfiguration configuration?? ILogger logger?? DbSettings dbSettings)
8 : base(configuration??logger??dbSettings)
9 {
10 this._dbSettings = dbSettings;
11 }
12
13 protected override void OnModelCreating(DbModelBuilder modelBuilder)
14 {
15 base.OnModelCreating(modelBuilder);
16 modelBuilder.Properties().Where(o => o.Name == this._dbSettings.RowVersionNname)
17 .Configure(o => o.IsConcurrencyToken().HasDatabaseGeneratedOption(DatabaseGeneratedOption.None));
18 base.SetInitializer<VersionDbContext>();
19 }
20
21 public override int SaveChanges()
22 {
23 this.ChangeTracker.DetectChanges();
24 var objectContext = ((IObjectContextAdapter)this).ObjectContext;
25 foreach (ObjectStateEntry entry in objectContext.ObjectStateManager.GetObjectStateEntries(EntityState.Modified | EntityState.Added))
26 {
27 var v = entry.Entity;
28 if (v != null)
29 {
30 var property = v.GetType().GetProperty(this._dbSettings.RowVersionNname);
31 if (property != null)
32 {
33 var value = Encoding.UTF8.GetBytes(Guid.NewGuid().ToString());
34 property.SetValue(v?? value);
35 }
36 }
37 }
38 return base.SaveChanges();
39 }
40 }
41 }
????4.???XUnit??Rhino.Mocks??SqlServerCe???е??????
????????ο?nopcommerce?е???????nopcommerce????NUnit??????NUnit?????XUnit???????Nuget??????????????GitHub???aspnet????????????XUnit??
1 namespace Example.Infrastructure.Test.Repository
2 {
3 public class CustomerPersistenceTest
4 {
5 private IRepository<T> GetRepository<T>() where T : class
6 {
7 string testDbName = "Data Source=" + (System.IO.Path.GetDirectoryName(System.Reflection.Assembly.GetExecutingAssembly().Location)) + @"\test.sdf;Persist Security Info=False";
8 var configuration = MockRepository.GenerateMock<IConfiguration>();
9 var logger = MockRepository.GenerateMock<ILogger>();
10 var repository = new EfRepository<T>(new SqlServerDbContext(configuration??logger??new DbSettings
11 {
12 DbConnectionFactory = new SqlCeConnectionFactory("System.Data.SqlServerCe.4.0")??
13 NameOrConnectionString = testDbName??
14 Debug = true??
15 UnitTest = true??
16 EntityMaps = new List<object> { new EntityTypeConfiguration<Customer>() }
17 }));
18 return repository;
19 }
20
21 [Fact]
22 public void SaveLoadCustomerTest()
23 {
24 var repository = this.GetRepository<Customer>();
25 repository.Add(new Customer { UserName = "test" });
26 repository.Commit();
27 var customer = repository.Query.FirstOrDefault(o => o.UserName == "test");
28 Assert.NotNull(customer);
29 }
30 }
31 }
????5.?????ASP.NET???????????????????DbContext???????????Request??Χ
1 namespace Example.Web
2 {
3 public class MvcApplication : System.Web.HttpApplication
4 {
5 protected void Application_Start()
6 {
7 ObjectFactory.Init();
8 ObjectFactory.AddSingleton<IConfiguration?? AppConfigAdapter>();
9 ObjectFactory.AddSingleton<ILogger?? Log4netAdapter>();
10 ObjectFactory.AddSingleton<DbSettings?? DbSettings>(new DbSettings { NameOrConnectionString = "SqlCeConnection"?? Debug = true });
11 ObjectFactory.AddScoped<IDbContext?? SqlServerDbContext>();
12 ObjectFactory.AddTransient(typeof(IRepository<>)?? typeof(EfRepository<>));
13 ObjectFactory.Build();
14 ObjectFactory.GetInstance<ILogger>().Information(String.Format("Start at {0}"??DateTime.Now));
15 AreaRegistration.RegisterAllAreas();
16 FilterConfig.RegisterGlobalFilters(GlobalFilters.Filters);
17 RouteConfig.RegisterRoutes(RouteTable.Routes);
18 BundleConfig.RegisterBundles(BundleTable.Bundles);
19 }
20
21 protected void Application_EndRequest()
22 {
23 ObjectFactory.Dispose();
24 }
25 }
26 }
?????????????????????StructureMap??HttpContextLifecycle????Request??Χ???????????????δ??????StructureMap??????У????????StructureMap.Web??????????HttpContextLifecycle??????Application_EndRequest????HttpContextLifecycle.DisposeAndClearAll()??????
???????????????????????漰???????????????????SPASVOС??(021-61079698-8054)?????????????????????????
??????
iOS???????mocha??chai??sinon??istanbul???????????????????????????????????????д?????Java????????7??????Android?????????Robolectric3.0????(?)???Kiwi?????????????????????????????????Python?????????????????????????????????????Controller????????д?????????10???????????????????Angular????????????Component??Directive??Pipe ???ServiceAndroid????????????????????????????????--Mockito??????iOS UnitTest???????Vue?????????????????
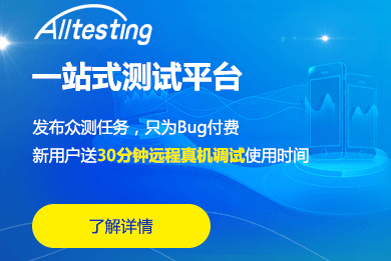
???·???
??????????????????
2023/3/23 14:23:39???д?ò??????????
2023/3/22 16:17:39????????????????????Щ??
2022/6/14 16:14:27??????????????????????????
2021/10/18 15:37:44???????????????
2021/9/17 15:19:29???·???????·
2021/9/14 15:42:25?????????????
2021/5/28 17:25:47??????APP??????????
2021/5/8 17:01:11????????
?????????App Bug???????????????????????Jmeter?????????QC??????APP????????????????app?????е????????jenkins+testng+ant+webdriver??????????????JMeter????HTTP???????Selenium 2.0 WebDriver ??????