??C#???????Щ??-??????
???????????? ???????[ 2016/4/29 13:35:02 ] ??????????????????? ???????
????2?????????
using System;
using System.Threading;
namespace Threading
{
class Program
{
public static void Thread1()
{
Console.WriteLine("Thread1 starts");
}
public static void Thread2(object data)
{
Console.WriteLine("Thread2 starts??para??{0}"?? data.ToString());
}
static void Main(string[] args)
{
var t1 = new Thread(Thread1);
t1.Start();
var t2 = new Thread(Thread2);
t2.Start("thread2");
Console.ReadKey();
}
}
}
????????
????Thread1 starts
????Thread2 starts??para??thread2
????3?????????
????sleep()?????Abort() ???????
?????????? threadabortexception ????????????????????????????????? finally ?飬????????? finally ??
using System;
using System.Threading;
namespace Threading
{
class Program
{
public static void Thread1()
{
Console.WriteLine("Thread1 starts");
Console.WriteLine("Thread1 Paused for 5 seconds");
Thread.Sleep(5000);
Console.WriteLine("Thread1 resumes");
}
static void Main(string[] args)
{
var t1 = new Thread(Thread1);
t1.Start();
Console.ReadKey();
}
}
}
using System;
using System.Threading;
namespace Threading
{
class Program
{
public static void Thread1()
{
try
{
Console.WriteLine("Thread1 starts");
for (int i = 0; i <= 10; i++)
{
Thread.Sleep(500);
Console.WriteLine(i);
}
Console.WriteLine("Thread1 Completed");
}
catch (ThreadAbortException ex)
{
Console.WriteLine("Thread1 Abort Exception");
}
finally
{
Console.WriteLine("Couldn't catch the Thread1 Exception");
}
}
static void Main(string[] args)
{
//?????????
var t1 = new Thread(Thread1);
t1.Start();
//????????2s
Thread.Sleep(2000);
//???t1?????
t1.Abort();
Console.ReadKey();
}
}
}
?????????????н????
???????????
??????????????У???????????仨??????????????????????????????????????????????ThreadPool??????????????????????????????????????????????????????????Timer????????????????
???????????????????????????????????????????????????????????????????????????????????????????????????????????????У????????????????????????????????????????У???????????????????????????????????????????????????????????????????????????????????????????
????????????????????????????????
????????????
using System;
using System.Threading;
namespace Threading
{
class Program
{
public static void Thread1(object data)
{
Console.WriteLine("Thread1 => {0}"??data.ToString());
}
static void Main(string[] args)
{
//???????????С
//?????????????????и???????????
//???????????????????? I/O ????????
ThreadPool.SetMaxThreads(3?? 3);
for (int i = 0; i < 10; i++)
{
//ThreadPool?????????????????
//ThreadPool.QueueUserWorkItem(new WaitCallback(Thread1)?? i);
ThreadPool.QueueUserWorkItem(Thread1?? i);
}
Console.WriteLine("Thread1 sleep");
Thread.Sleep(100000);
Console.WriteLine("Thread1 end");
Console.ReadKey();
}
}
}
??????
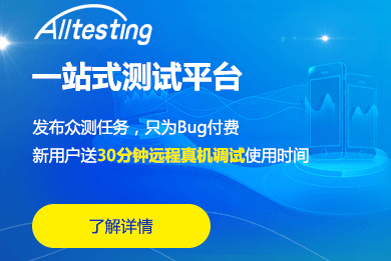
???·???
??????????????????
2023/3/23 14:23:39???д?ò??????????
2023/3/22 16:17:39????????????????????Щ??
2022/6/14 16:14:27??????????????????????????
2021/10/18 15:37:44???????????????
2021/9/17 15:19:29???·???????·
2021/9/14 15:42:25?????????????
2021/5/28 17:25:47??????APP??????????
2021/5/8 17:01:11