C++ ????????μ????????
???????????? ???????[ 2015/8/28 10:54:51 ] ??????????????????? ???????
????#include <cerrno>
????#include <cstdio>
????#include <cstdlib>
????#include <fstream>
????#include <iostream>
????#include <numeric>
????#include <unordered_map>
????#include <string>
????#include <vector>
????using std::accumulate;
????using std::cerr;
????using std::cout;
????using std::endl;
????using std::ifstream;
????using std::make_pair;
????using std::pair;
????using std::strerror;
????using std::string;
????using std::unordered_map;
????using std::vector;
????int word_count(const char *const file) noexcept(false);
????int main(int argc?? char *argv[]) {
????vector< pair<string?? int> > counts {};
????for (auto i = 1; i < argc; i += 1) {
????try {
????counts.push_back(make_pair(argv[i]?? word_count(argv[i])));
????} catch (const string& e) {
????cerr << e << endl;
????counts.push_back(make_pair(argv[i]?? -1));
????}
????}
????for (auto& result : counts) {
????cout << result.first << ": " << result.second << " words" << endl;
????}
????return 0;
????}
????int
????word_count(const char *const file) noexcept(false) {
????errno = 0;
????ifstream fp(file);
????{
????// Does fp.fail() preserve errno?
????int save_errno = errno;
????if (fp.fail()) {
????throw("Cannot open '" + string(file) + "': " + strerror(save_errno));
????}
????}
????unordered_map<string?? int> word_count {};
????string word;
????while (fp >> word) {
????word_count[word] += 1;
????}
????fp.close();
????return accumulate(
????word_count.cbegin()??
????word_count.cend()??
????0??
????[](int sum?? auto& el) { return sum += el.second; }
????);
????}
????20 ?д??????? #include ?? using ??????????????е??????????? using namespace std?????????????? std::… ???????????????????????С?
?????????????????п??ü?????????????????????????????????檔
???????????????????????????????????autovivification????
????unordered_map<string?? int> word_count {};
????string word;
????while (fp >> word) {
????word_count[word] += 1;
????}
???????????????? lambda ??????
????return accumulate(
????word_count.cbegin()??
????word_count.cend()??
????0??
????[](int sum?? auto& el) { return sum += el.second; }
????);
??????????accumulate ??????????????? 0????????????????????????????????????????????word_count???????????
?????????????ò??????????????Щ?????????????????? Microsoft Visual C++ 2015 RC ??????????????????????????????????·????
????????????
???????????ж??????????? boost libraries ????????????????????????????????????????????????? Perl ?? CPAN ?????????????д?????κε?????????е????????????????
???????磬??????????????????????????????? Excel ?????????????? Excel ??????????????? clang??g++ ?? cl ???????????????????????
?????????????л??????????????????????????Щ????????????????????????????????????д C++ ???????????????
????????????????????????????????о??????
???????????????????????漰???????????????????SPASVOС??(021-61079698-8054)?????????????????????????
??????
??C++????????????C++ lvalue??rvalueC++11????????C++???????????????C++?е?????????????????C++?????????C++???Windows????λ??C/C++???????????????????JAVA??C??C++??????????c++??python???????????????????????????????C++???????C++?е????????C++????????????????C++ ???????????????C++?????????????????????C++????????????
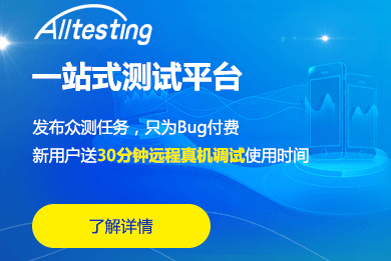
???·???
??????????????????
2023/3/23 14:23:39???д?ò??????????
2023/3/22 16:17:39????????????????????Щ??
2022/6/14 16:14:27??????????????????????????
2021/10/18 15:37:44???????????????
2021/9/17 15:19:29???·???????·
2021/9/14 15:42:25?????????????
2021/5/28 17:25:47??????APP??????????
2021/5/8 17:01:11????????
?????????App Bug???????????????????????Jmeter?????????QC??????APP????????????????app?????е????????jenkins+testng+ant+webdriver??????????????JMeter????HTTP???????Selenium 2.0 WebDriver ??????