??C#?Json????Json????????????
???????????? ???????[ 2015/7/2 14:10:08 ] ??????????????? C#
????ps:????????
1 using System;
2 using System.Collections.Generic;
3 using System.Linq;
4 using System.Text;
5 using Microsoft.JScript;
6
7 namespace Common
8 {
9 /// <summary>
10 /// Json?????zhuanh
11 /// </summary>
12 public class JsonHelper : IHelper
13 {
14 /// <summary>
15 /// ??????get set
16 /// </summary>
17 private bool isAddGetSet = false;
18
19 /// <summary>
20 /// ???????????
21 /// </summary>
22 private List<AutoClass> dataList = new List<AutoClass>();
23
24 public JsonHelper()
25 {
26 }
27
28 public JsonHelper(bool isAddGetSet)
29 {
30 this.isAddGetSet = isAddGetSet;
31 }
32
33 /// <summary>
34 /// ??????????????
35 /// </summary>
36 /// <param name="jsonStr"></param>
37 /// <returns></returns>
38 public string GetClassString(string jsonStr)
39 {
40 Microsoft.JScript.Vsa.VsaEngine ve = Microsoft.JScript.Vsa.VsaEngine.CreateEngine();
41 var m = Microsoft.JScript.Eval.JScriptEvaluate("(" + jsonStr + ")"?? ve);
42
43 int index = 0;
44 var result = GetDicType((JSObject)m?? ref index);
45
46 StringBuilder content = new StringBuilder();
47 foreach (var item in dataList)
48 {
49 content.AppendFormat(" public class {0} "?? item.CLassName);
50 content.AppendLine(" {");
51 foreach (var model in item.Dic)
52 {
53 if (isAddGetSet)
54 {
55 content.AppendFormat(" public {0} {1}"?? model.Value?? model.Key);
56 content.Append(" { get; set; } ");
57 }
58 else
59 {
60 content.AppendFormat(" public {0} {1}; "?? model.Value?? model.Key);
61 }
62
63 content.AppendLine();
64 }
65
66 content.AppendLine(" }");
67 content.AppendLine();
68 }
69
70 return content.ToString();
71 }
72
73 /// <summary>
74 /// ????????????????
75 /// </summary>
76 /// <param name="type"></param>
77 /// <returns></returns>
78 private string GetTypeString(Type type)
79 {
80 if (type == typeof(int))
81 {
82 return "int";
83 }
84 else if (type == typeof(bool))
85 {
86 return "bool";
87 }
88 else if (type == typeof(Int64))
89 {
90 return "long";
91 }
92 else if (type == typeof(string))
93 {
94 return "string";
95 }
96 else if (type == typeof(List<string>))
97 {
98 return "List<string>";
99 }
100 else if (type == typeof(List<int>))
101 {
102 return "List<int>";
103 }
104 else
105 {
106 return "string";
107 }
108 }
109
110 /// <summary>
111 /// ??????????
112 /// </summary>
113 /// <returns></returns>
114 private string GetDicType(JSObject jsObj?? ref int index)
115 {
116 AutoClass classInfo = new AutoClass();
117
118 var model = ((Microsoft.JScript.JSObject)(jsObj)).GetMembers(System.Reflection.BindingFlags.GetField);
119 foreach (Microsoft.JScript.JSField item in model)
120 {
121 string name = item.Name;
122 Type type = item.GetValue(item).GetType();
123 if (type == typeof(ArrayObject))
124 {
125 // ????
126 string typeName = GetDicListType((ArrayObject)item.GetValue(item)?? ref index);
127 if (!string.IsNullOrEmpty(typeName))
128 {
129 classInfo.Dic.Add(name?? typeName);
130 }
131 }
132 else if (type == typeof(JSObject))
133 {
134 // ????????
135 string typeName = GetDicType((JSObject)item.GetValue(item)?? ref index);
136 if (!string.IsNullOrEmpty(typeName))
137 {
138 classInfo.Dic.Add(name?? typeName);
139 }
140 }
141 else
142 {
143 classInfo.Dic.Add(name?? GetTypeString(type));
144 }
145 }
146
147 index++;
148 classInfo.CLassName = "Class" + index;
149 dataList.Add(classInfo);
150 return classInfo.CLassName;
151 }
152
153 /// <summary>
154 /// ???????????
155 /// </summary>
156 /// <param name="jsArray"></param>
157 /// <param name="index"></param>
158 /// <returns></returns>
159 private string GetDicListType(ArrayObject jsArray?? ref int index)
160 {
161 string name = string.Empty;
162 if ((int)jsArray.length > 0)
163 {
164 var item = jsArray[0];
165 var type = item.GetType();
166 if (type == typeof(JSObject))
167 {
168 name = "List<" + GetDicType((JSObject)item?? ref index) + ">";
169 }
170 else
171 {
172 name = "List<" + GetTypeString(type) + ">";
173 }
174 }
175
176 return name;
177 }
178 }
179
180 public class AutoClass
181 {
182 public string CLassName { get; set; }
183
184 private Dictionary<string?? string> dic = new Dictionary<string?? string>();
185
186 public Dictionary<string?? string> Dic
187 {
188 get
189 {
190 return this.dic;
191 }
192 set
193 {
194 this.dic = value;
195 }
196 }
197 }
198 }
???????÷????
????1 JsonHelper helper = new JsonHelper(true);
????2 try
????3 {
????4 this.txtOutPut.Text = helper.GetClassString("json?????");
????5 }
????6 catch
????7 {
????8 this.txtOutPut.Text = "?????????????淶...";
????9 }
?????????dudu???????????????????????????http://www.51debug.com/tool/JsonToCharpCode.aspx
???????????????????????漰???????????????????SPASVOС??(021-61079698-8054)?????????????????????????
??????
???????C#?е?StringC# Socket???????????????????C#?????????????????????????C#???????C#????????????Log4net??????δ????C#?????????????C#?????????????????C#???????????????C#????????????????C#7 ?е?Tuple??????C#??TypeScript - GeneratorC# ?????????細(xì)??????????C#?е???н???C#??MySQL?????????μ??Ч?????д??C#????C++??dll???C#??VS2010????е??????
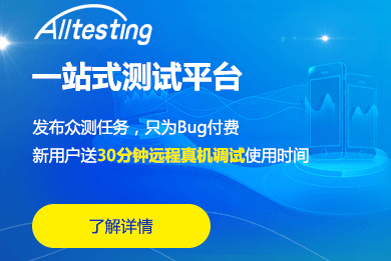
???·???
??????????????????
2023/3/23 14:23:39???д?ò??????????
2023/3/22 16:17:39????????????????????Щ??
2022/6/14 16:14:27??????????????????????????
2021/10/18 15:37:44???????????????
2021/9/17 15:19:29???·???????·
2021/9/14 15:42:25?????????????
2021/5/28 17:25:47??????APP??????????
2021/5/8 17:01:11????????
?????????App Bug???????????????????????Jmeter?????????QC??????APP????????????????app?????е????????jenkins+testng+ant+webdriver??????????????JMeter????HTTP???????Selenium 2.0 WebDriver ??????