?????????????????????ASP.NET?????????????
???????????? ???????[ 2015/4/16 14:24:43 ] ????????.NET ???? ???? ???????
???????????????????????????????????????????????????.??????????????????????????????????????Щ?????????????????“???”???????ο??????
???????????????????
?????????????Bit.ly??????????????????????????????????????????????????????????????????????????????????????????????????????????????????????????о?????????????????????????
???????????????????????1344573490??????????????????????????????????????????????????????????????????????????????????????????????????????????????????????????????????????????????????????????????????????????????洢?????ASP.NET???????У???????????????????????????????????????????
????public static class ScopedReferenceMap
????{
????private const int Buffer=32;
????///<summary>
????///Extension method to retrieve a public facing indirect value
????///</summary>
????///<typeparam name="T"></typeparam>
????///<param name="value"></param>
????///<returns></returns>
????public static string GetIndirectReference<T>(this T value)
????{
????//Get a converter to convert value to string
????var converter=TypeDescriptor.GetConverter(typeof(T));
????if(!converter.CanConvertTo(typeof(string)))
????{
????throw new ApplicationException("Can't convert value to string");
????}
????var directReference=converter.ConvertToString(value);
????return CreateOrAddMapping(directReference);
????}
????///<summary>
????///Extension method to retrieve the direct value from the user session
????///if it doesn't exists??the session has ended or this is possibly an attack
????///</summary>
????///<param name="indirectReference"></param>
????///<returns></returns>
????public static string GetDirectReference(this string indirectReference)
????{
????var map=HttpContext.Current.Session["RefMap"];
????if(map==null)throw new ApplicationException("Can't retrieve direct reference map");
????return((Dictionary<string??string>)map)[indirectReference];
????}
????private static string CreateOrAddMapping(string directReference)
????{
????var indirectReference=GetUrlSaveValue();
????var map=
????(Dictionary<string??string>)HttpContext.Current.Session["RefMap"]??
????new Dictionary<string??string>();
????//If we have it??return it.
????if(map.ContainsKey(directReference))return map[directReference];
????map.Add(directReference??indirectReference);
????map.Add(indirectReference??directReference);
????HttpContext.Current.Session["RefMap"]=map;
????return indirectReference;
????}
????private static string GetUrlSaveValue()
????{
????var csprng=new RNGCryptoServiceProvider();
????var buffer=new Byte[Buffer];
????//generate the random indirect value
????csprng.GetBytes(buffer);
????//base64 encode the random indirect value to a URL safe transmittable value
????return HttpServerUtility.UrlTokenEncode(buffer);
????}
????}
?????????????????????????????ScopedReferenceMap????????????????????????????????????п???1344573490?????Xvqw2JEm84w1qqLN1vE5XZUdc7BFqarB0????????ν???????á?
??????????????????????????????????????????????????????????е??????ú???????????????????????????????????????????????????????????????????????????????о??????????????????????м???????????
??????????????????κ?????????????????????????磺
????AccountNumber=accountNumber.GetIndirectReference();//create an indirect reference
?????????????????????URL??????????????????????????????
??????????????????accountNumber?????????????????????????????????μ????????
????[HttpGet]
????public ActionResult Details(string accountNumber)
????{
????//get direct reference
????var directRefstr=accountNumber.GetDirectReference();
????var accountNum=Convert.ToInt64(directRefstr);
????Account account=_accountRepository.Find(accountNum);
????//Verify authorization
????if(account.UserId!=User.Identity.GetUserId())
????{
????return new HttpUnauthorizedResult("User is not Authorized.");
????}
????//…
?????????????????????????У????ASP.NET???????л????????????????????????????????????????????????????????????????????????
???????????????????????????????????????????????????????????????????????????Щ?????????????????????????????????????????????????ASP.NET?????????????????????????????????????????????????????????Щ????????????????????Representational State Transfer?????????????糬y????????????????????????????????????????????????Щ??????ɡ?
????HATEOAS Gonna Hate
??????????????????????????е????????????????????????????????????request????????????????y???????????URLs)??response??????????????????web????????????????????????
??????????????????????????????REST?????????????????????y???????ó???????HATEOAS?????檔????仰??????HATEOAS????????????????????????????????????????HTTP?????????y????????????????????REST????????????????????????REST??HATEOAS???????????????????????????REST?????HATEOAS??????????????????????
????????????????????????????ò?????URL????????HATEOAS??????????????????????URL(???н??????????URL)??????к?????????????????????????URL????????????????????????????????????????????????????????????????
?????????????????
?????????????????????????URL???????????????Щ???????????????????????????????δ??????????????????????????????????????????????????????????????????????????????????????????????????????????????????????????????????t?????????????????????
???????????????B2B?????????????????????????????VIP?????????????????????????????????????????????????VIP?????????y?????????????????VIP??????????????????????????????????????п???VIP??????y???????
????????????????У????????????????????????????VIP???URL?е?VIP???ID???????????????????????????????????????ID??
?????????https://AppCore.com/business/Acme/VIP/Products/99933
??????????????????????????????????????????????????????????????????????ID???????????
????????????????????????????????????????????API???????????????????????????????????????????????????????????????????????????????
????public static class StaticReferenceMap
????{
????public const int KeySize=128;//bits
????public const int IvSize=16;//bytes
????public const int OutputByteSize=KeySize/8;
????private static readonly byte[]Key;
????static StaticReferenceMap()
????{
????Key=//pull 128 bit key in
????}
????///<summary>
????///Generates an encrypted value using symmetric encryption.
????///This is utilizing speed over strength due to the limit of security through obscurity
????///</summary>
????///<typeparam name="T">Primitive types only</typeparam>
????///<param name="value">direct value to be encrypted</param>
????///<returns>Encrypted value</returns>
????public static string GetIndirectReferenceMap<T>(this T value)
????{
????//Get a converter to convert value to string
????var converter=TypeDescriptor.GetConverter(typeof(T));
????if(!converter.CanConvertTo(typeof(string)))
????{
????throw new ApplicationException("Can't convert value to string");
????}
????//Convert value direct value to string
????var directReferenceStr=converter.ConvertToString(value);
????//encode using UT8
????var directReferenceByteArray=Encoding.UTF8.GetBytes(directReferenceStr);
????//Encrypt and return URL safe Token string which is the indirect reference value
????var urlSafeToken=EncryptDirectReferenceValue<T>(directReferenceByteArray);
????return urlSafeToken;
????}
????///<summary>
????///Give a encrypted indirect value??will decrypt the value and
????///return the direct reference value
????///</summary>
????///<param name="indirectReference">encrypted string</param>
????///<returns>direct value</returns>
????public static string GetDirectReferenceMap(this string indirectReference)
????{
????var indirectReferenceByteArray=
????HttpServerUtility.UrlTokenDecode(indirectReference);
????return DecryptIndirectReferenceValue(indirectReferenceByteArray);
????}
????private static string EncryptDirectReferenceValue<T>(byte[]directReferenceByteArray)
????{
????//IV needs to be a 16 byte cryptographic stength random value
????var iv=GetRandomValue();
????//We will store both the encrypted value and the IV used-IV is not a secret
????var indirectReferenceByteArray=new byte[OutputByteSize+IvSize];
????using(SymmetricAlgorithm algorithm=GetAlgorithm())
????{
????var encryptedByteArray=
????GetEncrptedByteArray(algorithm??iv??directReferenceByteArray);
????Buffer.BlockCopy(
????encryptedByteArray??0??indirectReferenceByteArray??0??OutputByteSize);
????Buffer.BlockCopy(iv??0??indirectReferenceByteArray??OutputByteSize??IvSize);
????}
????return HttpServerUtility.UrlTokenEncode(indirectReferenceByteArray);
????}
????private static string DecryptIndirectReferenceValue(
????byte[]indirectReferenceByteArray)
????{
????byte[]decryptedByteArray;
????using(SymmetricAlgorithm algorithm=GetAlgorithm())
????{
????var encryptedByteArray=new byte[OutputByteSize];
????var iv=new byte[IvSize];
????//separate off the actual encrypted value and the IV from the byte array
????Buffer.BlockCopy(
????indirectReferenceByteArray??
????0??
????encryptedByteArray??
????0??
????OutputByteSize);
????Buffer.BlockCopy(
????indirectReferenceByteArray??
????encryptedByteArray.Length??
????iv??
????0??
????IvSize);
????//decrypt the byte array using the IV that was stored with the value
????decryptedByteArray=GetDecryptedByteArray(algorithm??iv??encryptedByteArray);
????}
????//decode the UTF8 encoded byte array
????return Encoding.UTF8.GetString(decryptedByteArray);
????}
????private static byte[]GetDecryptedByteArray(
????SymmetricAlgorithm algorithm??byte[]iv??byte[]valueToBeDecrypted)
????{
????var decryptor=algorithm.CreateDecryptor(Key??iv);
????return decryptor.TransformFinalBlock(
????valueToBeDecrypted??0??valueToBeDecrypted.Length);
????}
????private static byte[]GetEncrptedByteArray(
????SymmetricAlgorithm algorithm??byte[]iv??byte[]valueToBeEncrypted)
????{
????var encryptor=algorithm.CreateEncryptor(Key??iv);
????return encryptor.TransformFinalBlock(
????valueToBeEncrypted??0??valueToBeEncrypted.Length);
????}
????private static AesManaged GetAlgorithm()
????{
????var aesManaged=new AesManaged
????{
????KeySize=KeySize??
????Mode=CipherMode.CBC??
????Padding=PaddingMode.PKCS7
????};
????return aesManaged;
????}
????private static byte[]GetRandomValue()
????{
????var csprng=new RNGCryptoServiceProvider();
????var buffer=new Byte[16];
????//generate the random indirect value
????csprng.GetBytes(buffer);
????return buffer;
????}
????}
???????????????API??????????ScopedReferenceMap???????????仯????????????У??????????.NET?о???128λ?????AesManaged?????????????????????IV????????????????????е??Щ?????????????????????????????????????????????
????AesManaged??????????FIPS???170????????AesCryptoServiceProvider
????128λ?????????????????????4?Σ??????????256λ?????С
???????????????????????????IV?????????????????????????????????????е????????С???????????????????????????????????????????????????????????????????????????????κ??????????????????洢?????????????????????????????????????????????????????н????????á?
??????????????????????????????????????????????????????????????????
?????????????????????????????????????????????????????????????????????AE???????????????????????????????????????????????????????????????????????????Stan Drapkin???????????????????????????????
?????????????????????????????????е???????????????????“????”?????????????????Щ????????????????????????.NET????????????????????????
?????μ???Щ
????????????????????????????????????????????????????????????????????????????δ????????
??????????????????????????????????????????????????????????????????????????????????????????????????????????????????????????????м???????????????????????????????????.NET?????????????????????????????????
?????????????ò????????????????????????????????????????е?????ü??????????????
????????????????????????????????????????????????????????????????????????????????????????????????????????????
???????
?????????????????????????漰????????????????????????????????????δ?????????????Σ??????????????ü?????????????????й??????????????????????????????Щ??????????????????????????????????????????????ü?????????????????????????
??????
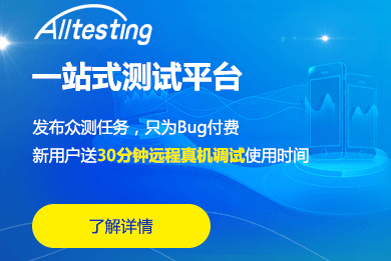
???·???
??????????????????
2023/3/23 14:23:39???д?ò??????????
2023/3/22 16:17:39????????????????????Щ??
2022/6/14 16:14:27??????????????????????????
2021/10/18 15:37:44???????????????
2021/9/17 15:19:29???·???????·
2021/9/14 15:42:25?????????????
2021/5/28 17:25:47??????APP??????????
2021/5/8 17:01:11