????Java??????÷?????
???????????? ???????[ 2015/3/18 14:05:59 ] ????????Java ??????? ?????
??????????????
????/*
????*????????????
????*???????????????????
????*/
????public class ThreadDemo3{
????private static boolean flags=false;
????public static void main(String[]args){
????class Person{
????public String name;
????private String gender;
????public void set(String name??String gender){
????this.name=name;
????this.gender=gender;
????}
????public void get(){
????System.out.println(this.name+"...."+this.gender);
????}
????}
????final Person p=new Person();
????new Thread(new Runnable(){
????public void run(){
????int x=0;
????while(true){
????synchronized(p){
????if(flags)
????try{
????p.wait();
????}catch(InterruptedException e){
????//TODO Auto-generated catch block
????e.printStackTrace();
????};
????if(x==0){
????p.set("????"??"??");
????}else{
????p.set("lili"??"nv");
????}
????x=(x+1)%2;
????flags=true;
????p.notifyAll();
????}
????}
????}
????}).start();
????new Thread(new Runnable(){
????public void run(){
????while(true){
????synchronized(p){
????if(!flags)
????try{
????p.wait();
????}catch(InterruptedException e){
????//TODO Auto-generated catch block
????e.printStackTrace();
????};
????p.get();
????flags=false;
????p.notifyAll();
????}
????}
????}
????}).start();
????}
????}
????????????????
????public class ThreadDemo4{
????private static boolean flags=false;
????public static void main(String[]args){
????class Goods{
????private String name;
????private int num;
????public synchronized void produce(String name){
????if(flags)
????try{
????wait();
????}catch(InterruptedException e){
????//TODO Auto-generated catch block
????e.printStackTrace();
????}
????this.name=name+"????"+num++;
????System.out.println("??????...."+this.name);
????flags=true;
????notifyAll();
????}
????public synchronized void consume(){
????if(!flags)
????try{
????wait();
????}catch(InterruptedException e){
????//TODO Auto-generated catch block
????e.printStackTrace();
????}
????System.out.println("??????******"+name);
????flags=false;
????notifyAll();
????}
????}
????final Goods g=new Goods();
????new Thread(new Runnable(){
????public void run(){
????while(true){
????g.produce("???");
????}
????}
????}).start();
????new Thread(new Runnable(){
????public void run(){
????while(true){
????g.consume();
????}
????}
????}).start();
????}
????}
???????????????2
????public class ThreadDemo4{
????private static boolean flags=false;
????public static void main(String[]args){
????class Goods{
????private String name;
????private int num;
????public synchronized void produce(String name){
????while(flags)
????try{
????wait();
????}catch(InterruptedException e){
????//TODO Auto-generated catch block
????e.printStackTrace();
????}
????this.name=name+"????"+num++;
????System.out.println(Thread.currentThread().getName()+"??????...."+this.name);
????flags=true;
????notifyAll();
????}
????public synchronized void consume(){
????while(!flags)
????try{
????wait();
????}catch(InterruptedException e){
????//TODO Auto-generated catch block
????e.printStackTrace();
????}
????System.out.println(Thread.currentThread().getName()+"??????******"+name);
????flags=false;
????notifyAll();
????}
????}
????final Goods g=new Goods();
????new Thread(new Runnable(){
????public void run(){
????while(true){
????g.produce("???");
????}
????}
????}??"?????????").start();
????new Thread(new Runnable(){
????public void run(){
????while(true){
????g.produce("???");
????}
????}
????}??"?????????").start();
????new Thread(new Runnable(){
????public void run(){
????while(true){
????g.consume();
????}
????}
????}??"?????????").start();
????new Thread(new Runnable(){
????public void run(){
????while(true){
????g.consume();
????}
????}
????}??"?????????").start();
????}
????}
????/*
???????????????????******???????48049
???????????????????....???????48050
???????????????????******???????48050
???????????????????....???????48051
???????????????????******???????48051
???????????????????....???????48052
???????????????????******???????48052
???????????????????....???????48053
???????????????????******???????48053
???????????????????....???????48054
???????????????????******???????48054
???????????????????....???????48055
???????????????????******???????48055
????*/
???????????????????????漰???????????????????SPASVOС??(021-61079698-8054)?????????????????????????
??????
Java???????????Щ???????????????Java????????????????Java?б???Map????????Java Web???????????????Java??????????????д?????Java????????7???????????????????????(java .net ?????)???Java??????????Python??????Java webdriver??λ????????′????е?????Java??д??????????????????Java???????????????JavaScript????????????Java?????????????????? Java???????10??????????????Java?м????????????????java???????ü???????????м???????????????????
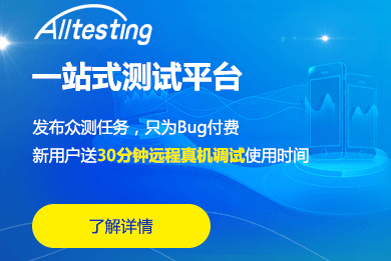
???·???
??????????????????
2023/3/23 14:23:39???д?ò??????????
2023/3/22 16:17:39????????????????????Щ??
2022/6/14 16:14:27??????????????????????????
2021/10/18 15:37:44???????????????
2021/9/17 15:19:29???·???????·
2021/9/14 15:42:25?????????????
2021/5/28 17:25:47??????APP??????????
2021/5/8 17:01:11????????
?????????App Bug???????????????????????Jmeter?????????QC??????APP????????????????app?????е????????jenkins+testng+ant+webdriver??????????????JMeter????HTTP???????Selenium 2.0 WebDriver ??????