C++????????????
???????????? ???????[ 2015/1/30 11:37:58 ] ????????C++ ????? ????
????ExpressManager.cpp
#include "stdafx.h"
#include "ExpressManager.h"
// pass the express manager
// @ car the pass car
// @ passCharge the charge for passing
float ExpressManager::Pass( const struct s_Car* car??float passCharge??float& rCharge )
{
if( car !=NULL )
{
float need = Caculate( car );
_tempCharge+=passCharge;
if( _tempCharge >= need )
{
_passCharge += need;
rCharge = _tempCharge - need;
_tempCharge = 0;
return true;
}
else
{
printf( "Fail to pass ! less %0.2f
"??need-_tempCharge );
return false;
}
}
else
{
printf("Fail to pass Null data!
");
}
return false;
}
// show the charge for passing
// @ car the pass car
float ExpressManager::ShowCharge( const struct s_Car* car ) const
{
if( car != NULL )
{
return Caculate( car );
}
else
{
printf("Fail to show Null data!
");
return 0.0f;
}
}
void ExpressManager::PassCar()
{
struct s_Car temp={0};
float pCharge=0.0f;
float rCharge=0.0f;
printf("plz??input car info! e. type trip
");
if( scanf_s("%d %f"??&temp.type??&temp.trip )!= EOF )
{
temp.passCharge = ShowCharge( &temp );
printf("plz pay %0.2f for passing
"??temp.passCharge );
while(true)
{
if( scanf_s( "%f"??&pCharge ) != EOF )
{
if( Pass( &temp??pCharge??rCharge ) )
{
if( rCharge==0.0f)
{
printf("congratulation! see you next time!
");
}
else
{
printf("congratulation! payback you %0.2f see you next time!
"??rCharge);
}
break;
}
}
}
}
}
void ExpressManager::PassTest()
{
int cmd = 0;
while(true)
{
printf("welcome to %s
"??_name);
printf(" 'p' - pass the car!
");
printf(" 's' - show the total charge!
");
printf(" 'q' - Exit!
");
fflush(stdin);
if( (cmd = getchar()) !=EOF )
{
if( cmd == 'q' ) break;
else if( cmd == 's' )
{
ShowTotal();
}
else if( cmd == 'p' )
{
PassCar();
}
}
}
}
???????????
1 #include "ExpressManager.h"
2 #include <stdio.h>
3
4 int main(int argc?? char* argv[])
5 {
6 ExpressManager *Express1=new ExpressManager("G2023");
7
8 Express1->PassTest();
9
10 return 0;
11 }
??????
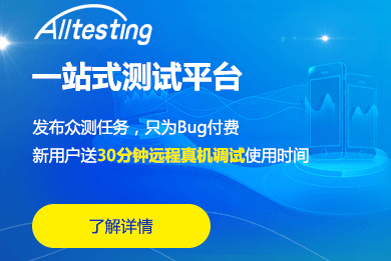
???·???
??????????????????
2023/3/23 14:23:39???д?ò??????????
2023/3/22 16:17:39????????????????????Щ??
2022/6/14 16:14:27??????????????????????????
2021/10/18 15:37:44???????????????
2021/9/17 15:19:29???·???????·
2021/9/14 15:42:25?????????????
2021/5/28 17:25:47??????APP??????????
2021/5/8 17:01:11