Java???????????
???????????? ???????[ 2015/10/10 13:32:32 ] ??????????????? ???????????
??????????????
?????????????????Ч????????????????????????????????????????????????????????????????????????????????????????????????????????????InvocationHandler?У??????????????????е??????
????InvocationHandler?????
????import java.lang.reflect.InvocationHandler;
????import java.lang.reflect.Method;
????public class PerformanceInterceptor implements InvocationHandler {
????private Object proxied;
????public PerformanceInterceptor(Object proxied) {
????this.proxied = proxied;
????}
????@Override
????public Object invoke(Object proxy?? Method method?? Object[] args) throws Throwable {
????long startTime = System.currentTimeMillis();
????Object obj = method.invoke(proxied?? args);
????long endTime = System.currentTimeMillis();
????System.out.println("Method " + method.getName() + " execution time: " + (endTime - startTime) * 1.0 / 1000 + "s");
????return obj;
????}
????}
??????????
????import java.lang.reflect.InvocationHandler;
????import java.lang.reflect.Proxy;
????import org.junit.Test;
????public class PerformanceInterceptorTest {
????@Test
????public void testInvoke() {
????UserDao userDao = new UserDaoImpl();
????Class<?> cls = userDao.getClass();
????InvocationHandler handler = new PerformanceInterceptor(userDao);
????UserDao proxy = (UserDao) Proxy.newProxyInstance(cls.getClassLoader()?? cls.getInterfaces()?? handler);
????proxy.addUser(new User("tom"));
????proxy.deleteUser("tom");
????proxy.updateUser("tom");
????}
????}
??????????
????add user named Tom...
????Method addUser execution time: 1.0s
????delete user named tom...
????Method deleteUser execution time: 1.5s
????update user named tom...
????Method updateUser execution time: 2.0s
???????????
??????????????????????????к???н????????Щ?????????????????????з????????Щ???????м???????????д??????????
????InvocationHandler?????
import java.io.File;
import java.io.FileWriter;
import java.io.IOException;
import java.lang.reflect.InvocationHandler;
import java.lang.reflect.Method;
import java.text.DateFormat;
import java.text.SimpleDateFormat;
public class LogInterceptor implements InvocationHandler {
private Object proxied;
public static final String path = "run.log";
public LogInterceptor(Object proxied) {
this.proxied = proxied;
}
public String beforeMethod(Method method) {
return getFormatedTime() + " Method:" + method.getName() + " start running ";
}
public String afterMethod(Method method) {
return getFormatedTime() + " Method:" + method.getName() + " end running ";
}
@Override
public Object invoke(Object proxy?? Method method?? Object[] args) throws Throwable {
write(path?? beforeMethod(method));
Object object = method.invoke(proxied?? args);
write(path?? afterMethod(method));
return object;
}
public String getFormatedTime() {
DateFormat formater = new SimpleDateFormat("yyyy-MM-dd hh:mm:ss");
return formater.format(System.currentTimeMillis());
}
public void write(String path?? String content) {
FileWriter writer = null;
try {
writer = new FileWriter(new File(path)?? true);
writer.write(content);
writer.flush();
} catch (IOException e) {
e.printStackTrace();
} finally {
if(null != writer) {
try {
writer.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
}
}
??????????
????import java.lang.reflect.InvocationHandler;
????import java.lang.reflect.Proxy;
????import org.junit.Test;
????public class LogInterceptorTest {
????@Test
????public void testInvoke() {
????UserDao userDao = new UserDaoImpl();
????Class<?> cls = userDao.getClass();
????InvocationHandler handler = new LogInterceptor(userDao);
????UserDao proxy = (UserDao) Proxy.newProxyInstance(cls.getClassLoader()?? cls.getInterfaces()?? handler);
????proxy.addUser(new User("tom"));
????proxy.deleteUser("tom");
????proxy.updateUser("tom");
????}
????}
??????????
??????????????????run.log????????????£?
????2015-10-07 05:41:02 Method:addUser start running
????2015-10-07 05:41:03 Method:addUser end running
????2015-10-07 05:41:03 Method:deleteUser start running
????2015-10-07 05:41:05 Method:deleteUser end running
????2015-10-07 05:41:05 Method:updateUser start running
????2015-10-07 05:41:07 Method:updateUser end running
???????
????????Java????????????????????????????в???????????Java???????????????????????????????????κν???????д?????????????????????????cdlib??Java???????????????AOP????????????????????????????????????????????????Щ??????????????????????????????Щ?????????
???????????????????????漰???????????????????SPASVOС??(021-61079698-8054)?????????????????????????
??????
Java???????????Щ???????????????Java????????????????Java?б???Map????????Java Web???????????????Java??????????????д?????Java????????7???????????????????????(java .net ?????)???Java??????????Python??????Java webdriver??λ????????′????е?????Java??д??????????????????Java???????????????JavaScript????????????Java?????????????????? Java???????10??????????????Java?м????????????????java???????ü???????????м???????????????????
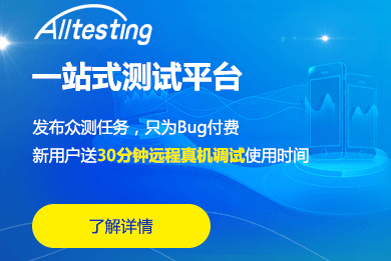
???·???
??????????????????
2023/3/23 14:23:39???д?ò??????????
2023/3/22 16:17:39????????????????????Щ??
2022/6/14 16:14:27??????????????????????????
2021/10/18 15:37:44???????????????
2021/9/17 15:19:29???·???????·
2021/9/14 15:42:25?????????????
2021/5/28 17:25:47??????APP??????????
2021/5/8 17:01:11????????
?????????App Bug???????????????????????Jmeter?????????QC??????APP????????????????app?????е????????jenkins+testng+ant+webdriver??????????????JMeter????HTTP???????Selenium 2.0 WebDriver ??????