C++????????÷????
???????????? ???????[ 2014/9/19 13:39:18 ] ??????????????? Net C++
???????????? std::auto_ptr ??????????“operator=”???????????????????????????????????????[1]???????????е????????
???????? std::auto_ptr ?t?????????????????????????????????
????void TestAutoPtr3() {
????std::auto_ptr<Simple> my_memory(new Simple(1));
????if (my_memory.get()) {
????my_memory.release();
????}
????}
??????н?????
????Simple: 1
??????????????????????????????????б?????????????“~Simple: 1”?????????й?????????????? my_memory ???????????????????? release() ?????????棬???????????й???????????????У????my_memory????????棬????????????????????黹?????????? my_memory ????????????黹????
??????????????????
void TestAutoPtr3() {
std::auto_ptr<Simple> my_memory(new Simple(1));
if (my_memory.get()) {
Simple* temp_memory = my_memory.release();
delete temp_memory;
}
}
??
void TestAutoPtr3() {
std::auto_ptr<Simple> my_memory(new Simple(1));
if (my_memory.get()) {
my_memory.reset(); // ??? my_memory ???????????
}
}
??????? std::auto_ptr ?? release() ????????ó??????????????????????? C++ ??????
???????std::auto_ptr ?????????????????????棬?????????????????
??????1?? ??????????“operator=”??????????????????????????
??????2?? ??? release() ???????????????????黹???????
??????3?? std::auto_ptr ?ò?????????????????????????д?????????????????
??????4?? ???? std::auto_ptr ??“operator=”???????????????????? std::vector ???????С?
??????5?? ……
?????????? std::auto_ptr ???????????????????????????????飬???????????????????????????????????????????????С??????????????
???????? std::auto_ptr ??????????????Щ?????????????? C++ ???????????????????? boost ?????????boost ????????????????????
????????????????????
????3??boost::scoped_ptr
????boost::scoped_ptr ???? boost ???????? namespace boost ?У????????? #include<boost/smart_ptr.hpp> ???????á?boost::scoped_ptr ?? std::auto_ptr ??????????????????????????????????boost::scoped_ptr ????????????????? std::auto_ptr ????????????
??????????????????????
void TestScopedPtr() {
boost::scoped_ptr<Simple> my_memory(new Simple(1));
if (my_memory.get()) {
my_memory->PrintSomething();
my_memory.get()->info_extend = "Addition";
my_memory->PrintSomething();
(*my_memory).info_extend += " other";
my_memory->PrintSomething();
my_memory.release(); // ???? error: scoped_ptr ??? release ????
std::auto_ptr<Simple> my_memory2;
my_memory2 = my_memory; // ???? error: scoped_ptr ??????? operator=????????????????
}
}
?????????????????????boost::scoped_ptr ??????? auto_ptr ?????????á???????? release() ????????????????????й???????Σ????? boost::scoped_ptr ??????????????????????????д“my_memory2 = my_memory”?????????????? std::auto_ptr ?????????????
???????? boost::scoped_ptr ???????????????????????????????????????????????????????????????????????????????????????????????????????????????μ? boost::shared_ptr??
????4??boost::shared_ptr
????boost::shared_ptr ???? boost ???????? namespace boost ?У????????? #include<boost/smart_ptr.hpp> ???????á?????????????? boost::scoped_ptr ???????????????????????????boost::shared_ptr ???????????????????????????????????????????????????ü?????boost::shared_ptr ???????????????????????
??????????????????????
void TestSharedPtr(boost::shared_ptr<Simple> memory) { // ?????????? reference (?? const reference)
memory->PrintSomething();
std::cout << "TestSharedPtr UseCount: " << memory.use_count() << std::endl;
}
void TestSharedPtr2() {
boost::shared_ptr<Simple> my_memory(new Simple(1));
if (my_memory.get()) {
my_memory->PrintSomething();
my_memory.get()->info_extend = "Addition";
my_memory->PrintSomething();
(*my_memory).info_extend += " other";
my_memory->PrintSomething();
}
std::cout << "TestSharedPtr2 UseCount: " << my_memory.use_count() << std::endl;
TestSharedPtr(my_memory);
std::cout << "TestSharedPtr2 UseCount: " << my_memory.use_count() << std::endl;
//my_memory.release();// ???? error: ?????shared_ptr ???? release ????
}
??????н?????
????Simple: 1
????PrintSomething:
????PrintSomething: Addition
????PrintSomething: Addition other
????TestSharedPtr2 UseCount: 1
????PrintSomething: Addition other
????TestSharedPtr UseCount: 2
????TestSharedPtr2 UseCount: 1
????~Simple: 1
????boost::shared_ptr ????????????á???????? release() ??????????????boost::shared_ptr ??????????????ü????????????????????????????boost::shared_ptr ??????????? use_count() ??????????? boost::shared_ptr ????????ü?????????н???????????????? TestSharedPtr2 ?????У????ü???? 1??????????????????????θ???????????TestSharedPtr ????????ü????2???? TestSharedPtr ????????ü????????? 1????????????????????????????boost::shared_ptr ????ò????????
??????????????????????????????????????????????????????飬???????????????
????5??boost::scoped_array
????boost::scoped_array ???? boost ???????? namespace boost ?У????????? #include<boost/smart_ptr.hpp> ???????á?
????boost::scoped_array ????????????????????? boost::scoped_ptr ???????????????????
??????????????????????
void TestScopedArray() {
boost::scoped_array<Simple> my_memory(new Simple[2]); // ?????????????????
if (my_memory.get()) {
my_memory[0].PrintSomething();
my_memory.get()[0].info_extend = "Addition";
my_memory[0].PrintSomething();
(*my_memory)[0].info_extend += " other"; // ???? error??scoped_ptr ??????? operator*
my_memory[0].release(); // ??????? release ????
boost::scoped_array<Simple> my_memory2;
my_memory2 = my_memory; // ???? error????????????? operator=
}
}
????boost::scoped_array ?????? boost::scoped_ptr ?????????????????????????????????????顣????boost::scoped_array ???????“operator*”???????????????????£???????? get() ?????????Щ??
????????????y? boost::shared_array ???????????ü?????????????????????????????
????6??boost::shared_array
????boost::shared_array ???? boost ???????? namespace boost ?У????????? #include<boost/smart_ptr.hpp> ???????á?
???????? boost::scoped_array ??????????????????????£??????????????????????????????????????? boost::shared_array???? boost::shared_ptr ????????????????ü?????
??????????????????????
void TestSharedArray(boost::shared_array<Simple> memory) { // ?????????? reference (?? const reference)
std::cout << "TestSharedArray UseCount: " << memory.use_count() << std::endl;
}
void TestSharedArray2() {
boost::shared_array<Simple> my_memory(new Simple[2]);
if (my_memory.get()) {
my_memory[0].PrintSomething();
my_memory.get()[0].info_extend = "Addition 00";
my_memory[0].PrintSomething();
my_memory[1].PrintSomething();
my_memory.get()[1].info_extend = "Addition 11";
my_memory[1].PrintSomething();
//(*my_memory)[0].info_extend += " other"; // ???? error??scoped_ptr ??????? operator*
}
std::cout << "TestSharedArray2 UseCount: " << my_memory.use_count() << std::endl;
TestSharedArray(my_memory);
std::cout << "TestSharedArray2 UseCount: " << my_memory.use_count() << std::endl;
}
??????н?????
????Simple: 0
????Simple: 0
????PrintSomething:
????PrintSomething: Addition 00
????PrintSomething:
????PrintSomething: Addition 11
????TestSharedArray2 UseCount: 1
????TestSharedArray UseCount: 2
????TestSharedArray2 UseCount: 1
????~Simple: 0
????~Simple: 0
?????? boost::shared_ptr ?????????????ü???????????????????????????
??????????????????????????? std::auto_ptr??boost::scoped_ptr??boost::shared_ptr??boost::scoped_array??boost::shared_array????????????????????????????????90% ????ù????????????????? 5 ????????????????????????????????????????????????????ɡ?
???????????????????????漰???????????????????SPASVOС??(021-61079698-8054)?????????????????????????
??????
??C++????????????C++ lvalue??rvalueC++11????????C++???????????????C++?е?????????????????C++?????????C++???Windows????λ??C/C++???????????????????JAVA??C??C++??????????c++??python???????????????????????????????C++???????C++?е????????C++????????????????C++ ???????????????C++?????????????????????C++????????????
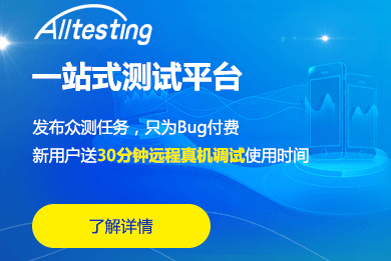
???·???
??????????????????
2023/3/23 14:23:39???д?ò??????????
2023/3/22 16:17:39????????????????????Щ??
2022/6/14 16:14:27??????????????????????????
2021/10/18 15:37:44???????????????
2021/9/17 15:19:29???·???????·
2021/9/14 15:42:25?????????????
2021/5/28 17:25:47??????APP??????????
2021/5/8 17:01:11????????
?????????App Bug???????????????????????Jmeter?????????QC??????APP????????????????app?????е????????jenkins+testng+ant+webdriver??????????????JMeter????HTTP???????Selenium 2.0 WebDriver ??????