C++11???????Щ????????
???????????? ???????[ 2014/9/10 11:10:26 ] ??????????????? ??
????c++11?????????Щ????????????Щ?????????????????д???????????????????о??Щ??????????????????????????????????????????ο?http://en.cppreference.com/w/cpp/algorithm??
???????????????????????ж????all_of??any_of??none_of??
????template< class InputIt?? class UnaryPredicate >
????bool all_of( InputIt first?? InputIt last?? UnaryPredicate p );
????template< class InputIt?? class UnaryPredicate >
????bool any_of( InputIt first?? InputIt last?? UnaryPredicate p );
????template< class InputIt?? class UnaryPredicate >
????bool none_of( InputIt first?? InputIt last?? UnaryPredicate p );
????all_of:???????[first?? last)????????е????????????ж??p?????е?????????????????true????????false??
????any_of?????????[first?? last)?????????????????????????ж??p????????????????????????true????????true??
????none_of?????????[first?? last)????????е??????????????ж??p?????е???????????????????true????????false??
???????????????????????
#include <iostream>
#include <algorithm>
#include <vector>
using namespace std;
int main()
{
vector<int> v = { 1?? 3?? 5?? 7?? 9 };
auto isEven = [](int i){return i % 2 != 0;
bool isallOdd = std::all_of(v.begin()?? v.end()?? isEven);
if (isallOdd)
cout << "all is odd" << endl;
bool isNoneEven = std::none_of(v.begin()?? v.end()?? isEven);
if (isNoneEven)
cout << "none is even" << endl;
vector<int> v1 = { 1?? 3?? 5?? 7?? 8?? 9 };
bool anyof = std::any_of(v1.begin()?? v1.end()?? isEven);
if (anyof)
cout << "at least one is even" << endl;
}
?????????
????all is odd
????none is odd
????at least one is even
???????????????????????find_if_not??????????find_if???????????????????????????????find_if????????find_if_not?????????????ж??????????ж?????????????????find_if_not??????????д?????ж????????????????á???????????????÷???
#include <iostream>
#include <algorithm>
#include <vector>
using namespace std;
int main()
{
vector<int> v = { 1?? 3?? 5?? 7?? 9??4 };
auto isEven = [](int i){return i % 2 == 0;};
auto firstEven = std::find_if(v.begin()?? v.end()?? isEven);
if (firstEven!=v.end())
cout << "the first even is " <<* firstEven << endl;
//??find_if???????????????????д???????????ж??
auto isNotEven = [](int i){return i % 2 != 0;};
auto firstOdd = std::find_if(v.begin()?? v.end()??isNotEven);
if (firstOdd!=v.end())
cout << "the first odd is " <<* firstOdd << endl;
//??find_if_not??????????????????????ж??
auto odd = std::find_if_not(v.begin()?? v.end()?? isEven);
if (odd!=v.end())
cout << "the first odd is " <<* odd << endl;
}
???????????
????the first even is 4
????the first odd is 1
????the first odd is 1
??????
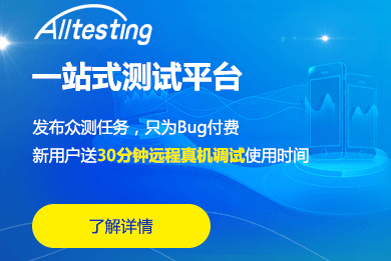
???·???
??????????????????
2023/3/23 14:23:39???д?ò??????????
2023/3/22 16:17:39????????????????????Щ??
2022/6/14 16:14:27??????????????????????????
2021/10/18 15:37:44???????????????
2021/9/17 15:19:29???·???????·
2021/9/14 15:42:25?????????????
2021/5/28 17:25:47??????APP??????????
2021/5/8 17:01:11