C++???dll???????????
???????????? ???????[ 2014/3/24 10:57:11 ] ????????DLL ??? ????
// ?????????????????ID??????ж????????????????????????????????ID
DWORD processNameToId(LPCTSTR lpszProcessName)
{
HANDLE hSnapshot = CreateToolhelp32Snapshot(TH32CS_SNAPPROCESS?? 0);
PROCESSENTRY32 pe;
pe.dwSize = sizeof(PROCESSENTRY32);
if( !Process32First(hSnapshot?? &pe) )
{
MessageBox( NULL??
"The frist entry of the process list has not been copyied to the buffer"??
"Notice"??
MB_ICONINFORMATION | MB_OK
);
return 0;
}
while( Process32Next(hSnapshot?? &pe) )
{
if( !strcmp(lpszProcessName?? pe.szExeFile) )
{
return pe.th32ProcessID;
}
}
return 0;
}
int main(int argc?? char* argv[])
{
// ???????????С
const DWORD dwThreadSize = 5 * 1024;
DWORD dwWriteBytes;
// ??????????????
enableDebugPriv();
// ???????????????????Сд???
std::cout << "Please input the name of target process !" << std::endl;
char szExeName[MAX_PATH] = { 0 };
std::cin >> szExeName;
DWORD dwProcessId = processNameToId(szExeName);
if( dwProcessId == 0 )
{
MessageBox( NULL??
"The target process have not been found !"??
"Notice"??
MB_ICONINFORMATION | MB_OK
);
return -1;
}
// ???????ID?????????
HANDLE hTargetProcess = OpenProcess(PROCESS_ALL_ACCESS?? FALSE?? dwProcessId);
if( !hTargetProcess )
{
MessageBox( NULL??
"Open target process failed !"??
"Notice"??
MB_ICONINFORMATION | MB_OK
);
return 0;
}
// ?????????????????忪?????洢????
// ????????????MEM_COMMIT?????????????PAGE_EXECUTE_READWRITE??汣??????
// ????庬????ο?MSDN?й???VirtualAllocEx???????????
void* pRemoteThread = VirtualAllocEx( hTargetProcess??
0??
dwThreadSize??
MEM_COMMIT ?? PAGE_EXECUTE_READWRITE);
if( !pRemoteThread )
{
MessageBox( NULL??
"Alloc memory in target process failed !"??
"notice"??
MB_ICONINFORMATION | MB_OK
);
return 0;
}
// ???????????DLL????
char szDll[256];
memset(szDll?? 0?? 256);
strcpy(szDll?? "E:\mydll.dll");
// ???????DLL????????????
if( !WriteProcessMemory( hTargetProcess??
pRemoteThread??
(LPVOID)szDll??
dwThreadSize??
0) )
{
MessageBox( NULL??
"Write data to target process failed !"??
"Notice"??
MB_ICONINFORMATION | MB_OK
);
return 0;
}
LPVOID pFunc = LoadLibraryA;
//???????????д??????
HANDLE hRemoteThread = CreateRemoteThread( hTargetProcess??
NULL??
0??
(LPTHREAD_START_ROUTINE)pFunc??
pRemoteThread??
0??
&dwWriteBytes);
if( !hRemoteThread )
{
MessageBox( NULL??
"Create remote thread failed !"??
"Notice"??
MB_ICONINFORMATION | MB_OK
);
return 0;
}
// ???LoadLibraryA???????
WaitForSingleObject(hRemoteThread?? INFINITE );
VirtualFreeEx(hTargetProcess?? pRemoteThread?? dwThreadSize?? MEM_COMMIT);
CloseHandle( hRemoteThread );
CloseHandle( hTargetProcess );
return 0;
}
??????
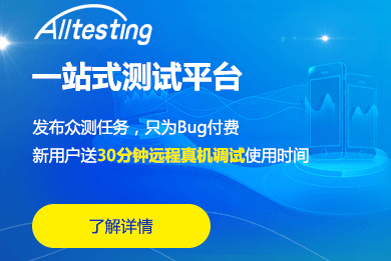
???·???
??????????????????
2023/3/23 14:23:39???д?ò??????????
2023/3/22 16:17:39????????????????????Щ??
2022/6/14 16:14:27??????????????????????????
2021/10/18 15:37:44???????????????
2021/9/17 15:19:29???·???????·
2021/9/14 15:42:25?????????????
2021/5/28 17:25:47??????APP??????????
2021/5/8 17:01:11