QML??C++????
???????????? ???????[ 2014/12/30 11:33:05 ] ????????C++ .NET
????????QML??C++??????????
?????????:
//numbercount.h
#ifndef NumberCount_H
#define NumberCount_H
#include <QObject>
#include <QVariant>
class NumberCount : public QObject
{
Q_OBJECT
public:
explicit NumberCount(QObject *parent = 0);
Q_INVOKABLE int getTotal();
signals:
void resetData(QVariant num);//???????qml??javascript ????
public slots:
int incre();
int decre();
void reset(int num);
private:
int count;
int total;
};
#endif // NumberCount_H
//==========================================================
//NumberCount.cpp
#include "numbercount.h"
#include <QDebug>
NumberCount::NumberCount(QObject *parent) :
QObject(parent)
{
this->total = 100;
}
int NumberCount::incre()
{
qDebug()<<"count=: "<< ++total;
count++;
return count;
}
int NumberCount::decre()
{
qDebug()<<"count=: "<< --total;
count--;
return count;
}
void NumberCount::reset(int num)
{
this->total = num;
count = 0;
emit resetData(QVariant(num));//????qml??javascript ????
}
int NumberCount::getTotal()
{
return total;
}
//==========================================================
//numbercount.qml
import Qt 4.7
Rectangle {
//signal resetData;
function resetData(text){
console.log("javascript resetData??????C++????!");
btn_decre.text = "????<font color='#0000FF'>(0)</font>";
btn_incre.text = "????<font color='#0000FF'>(0)</font>";
txt_show.text = "????:" + text;
txt_reset.text = "?????????:"+text;
}
width: 300
height: 300
radius: 20
anchors.fill: parent
Text {
id: txt_show
x: 150
y: 0
text : "????:" + numberCount.getTotal(100);
}
Rectangle{
x:100
y:50
width: 80
height: 25
Image{
anchors.fill: parent
width:parent.width
height: parent.width
source: "/ui/images/button.png"
}
Text {
id: btn_incre
anchors.centerIn: parent
text: "????"
MouseArea{
anchors.fill: parent
onClicked: {
var num = numberCount.incre();
parent.text = "????<font color='#0000FF'>(" + num +")</font>";
btn_decre.text = "????<font color='#0000FF'>("+ num+")</font>";
txt_show.text = "????:" + numberCount.getTotal();
}
}
}
}
Rectangle{
x:100
y:100
width: 80
height: 25
Image{
anchors.fill: parent
width:parent.width
height: parent.width
source: "/ui/images/button.png"
}
Text {
id: btn_decre
anchors.centerIn: parent
text: "????"
MouseArea{
anchors.fill: parent
onClicked: {
var num = numberCount.decre();
parent.text = "????<font color='#0000FF'>(" + num +")</font>";
btn_incre.text = "????<font color='#0000FF'>("+ num+")</font>";
txt_show.text = "????:" + numberCount.getTotal();
}
}
}
}
Rectangle{
x:100
y:150
width: 80
height: 25
Image{
anchors.fill: parent
width:parent.width
height: parent.width
source: "/ui/images/button.png"
}
Text {
id: btn_show
anchors.centerIn: parent
text: "????????"
MouseArea{
anchors.fill: parent
onClicked: {
numberCount.reset(200);
}
}
}
}
Text {
id: txt_reset
x: 100
y: 200
color: "#12ff12"
}
??????
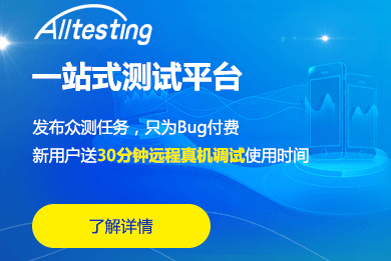
???·???
??????????????????
2023/3/23 14:23:39???д?ò??????????
2023/3/22 16:17:39????????????????????Щ??
2022/6/14 16:14:27??????????????????????????
2021/10/18 15:37:44???????????????
2021/9/17 15:19:29???·???????·
2021/9/14 15:42:25?????????????
2021/5/28 17:25:47??????APP??????????
2021/5/8 17:01:11