Java?????????????
???????????? ???????[ 2014/10/30 10:12:18 ] ????????Java ???????
????????????Demo?????
1 package com.red.test;
2
3 import net.sourceforge.pinyin4j.PinyinHelper;
4 import net.sourceforge.pinyin4j.format.HanyuPinyinCaseType;
5 import net.sourceforge.pinyin4j.format.HanyuPinyinOutputFormat;
6 import net.sourceforge.pinyin4j.format.HanyuPinyinToneType;
7 import net.sourceforge.pinyin4j.format.exception.BadHanyuPinyinOutputFormatCombination;
8
9 /**
10 * ???????????
11 * @author Red
12 */
13 public class PinyinDemo {
14 /**
15 * ????main????
16 * @param args
17 */
18 public static void main(String[] args) {
19 System.out.println(ToFirstChar("???????????").toUpperCase()); //?????????д
20 System.out.println(ToPinyin("???????????"));
21 }
22 /**
23 * ???????????????????
24 * @param chinese
25 * @return
26 */
27 public static String ToFirstChar(String chinese){
28 String pinyinStr = "";
29 char[] newChar = chinese.toCharArray(); //?????????
30 HanyuPinyinOutputFormat defaultFormat = new HanyuPinyinOutputFormat();
31 defaultFormat.setCaseType(HanyuPinyinCaseType.LOWERCASE);
32 defaultFormat.setToneType(HanyuPinyinToneType.WITHOUT_TONE);
33 for (int i = 0; i < newChar.length; i++) {
34 if (newChar[i] > 128) {
35 try {
36 pinyinStr += PinyinHelper.toHanyuPinyinStringArray(newChar[i]?? defaultFormat)[0].charAt(0);
37 } catch (BadHanyuPinyinOutputFormatCombination e) {
38 e.printStackTrace();
39 }
40 }else{
41 pinyinStr += newChar[i];
42 }
43 }
44 return pinyinStr;
45 }
46
47 /**
48 * ?????????
49 * @param chinese
50 * @return
51 */
52 public static String ToPinyin(String chinese){
53 String pinyinStr = "";
54 char[] newChar = chinese.toCharArray();
55 HanyuPinyinOutputFormat defaultFormat = new HanyuPinyinOutputFormat();
56 defaultFormat.setCaseType(HanyuPinyinCaseType.LOWERCASE);
57 defaultFormat.setToneType(HanyuPinyinToneType.WITHOUT_TONE);
58 for (int i = 0; i < newChar.length; i++) {
59 if (newChar[i] > 128) {
60 try {
61 pinyinStr += PinyinHelper.toHanyuPinyinStringArray(newChar[i]?? defaultFormat)[0];
62 } catch (BadHanyuPinyinOutputFormatCombination e) {
63 e.printStackTrace();
64 }
65 }else{
66 pinyinStr += newChar[i];
67 }
68 }
69 return pinyinStr;
70 }
71 }
??????
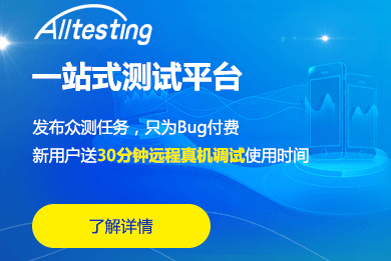
???·???
??????????????????
2023/3/23 14:23:39???д?ò??????????
2023/3/22 16:17:39????????????????????Щ??
2022/6/14 16:14:27??????????????????????????
2021/10/18 15:37:44???????????????
2021/9/17 15:19:29???·???????·
2021/9/14 15:42:25?????????????
2021/5/28 17:25:47??????APP??????????
2021/5/8 17:01:11