Java??????????
???????????? ???????[ 2015/6/17 11:04:15 ] ???????????????? ???????
???????????
????????????ζ?????????????????????????????????л??????????????ζ???????????????????????????????
????????????????????????????????????????????壬??????????任???????????????????????У???????????cpu?????io?????????Э??cpu?????????????????????д??????
????volatile
??????ó????????????????й????ж????????????????????????????????θ???????????????????????????鱻?ù???????ε?????????仯??
????CountDownLatch
??????ó????????????????????????????????????????????????????????????await()???????????????????????????????????countDown()??????
?????????????????
????1??????????????????????????(????????????)??
????2???????????д?????(??д???????????????????????????)??
????3????????????????????????д?????????????
???????????
?????????????????????????????for??????????????????????????????е?仯???????????????????д????????????????????????????????????????????sleep???
package org.wit.ff.ch2;
import java.io.BufferedWriter;
import java.io.File;
import java.io.FileWriter;
import java.io.IOException;
import java.util.Scanner;
import java.util.concurrent.BlockingQueue;
import java.util.concurrent.CountDownLatch;
import java.util.concurrent.LinkedBlockingQueue;
import java.util.concurrent.TimeUnit;
/**
*
* <pre>
* ???????????.
* </pre>
*
* @author F.Fang
* @version $Id: AsyncHandler.java?? v 0.1 2014??10??23?? ????11:37:54 F.Fang Exp $
*/
public class AsyncHandler {
/**
* ??????????.
*/
private CountDownLatch latch;
/**
* ?????????.
*/
private volatile boolean handleFinish;
/**
* ???д??????????.
*/
private volatile boolean sendFinish;
/**
* ????????.
*/
private BlockingQueue<String> queue;
private BufferedWriter bw;
public AsyncHandler(CountDownLatch latch) {
this.latch = latch;
/**
* ??????????.
*/
queue = new LinkedBlockingQueue<String>();
File file = new File("E:/hello.txt");
try {
bw = new BufferedWriter(new FileWriter(file));
} catch (IOException e) {
throw new RuntimeException(e);
}
}
public void handle() {
// ??????????????й????3s??????????.
new Thread() {
public void run() {
while (!handleFinish) {
try {
TimeUnit.SECONDS.sleep(3);
} catch (InterruptedException e1) {
// ????????.
}
String s = queue.peek();
if (s != null) {
queue.poll();
try {
bw.write(s);
bw.newLine();
} catch (IOException e) {
}
}
// ?????????????????????.
if (queue.isEmpty() && sendFinish) {
// ??????1->0
latch.countDown();
// ???????????.
handleFinish = true;
break;
}
}
}
}.start();
}
/**
*
* <pre>
* ?????????????????.
* </pre>
*
*/
public void sendFinish() {
sendFinish = true;
}
/**
*
* <pre>
* ??????.
* </pre>
*
*/
public void release() {
System.out.println("release!");
if (bw != null) {
try {
bw.close();
} catch (IOException e) {
// TODO ??????.
}
}
//??????queue = null????.
if (queue != null) {
queue.clear();
queue = null;
}
}
/**
*
* <pre>
* ?????з??????.
* </pre>
*
* @param text
*/
public void sendMsg(String text) {
if (text != null && !text.isEmpty()) {
queue.add(text);
}
}
public static void main(String[] args) {
CountDownLatch latch = new CountDownLatch(1);
AsyncHandler handler = new AsyncHandler(latch);
handler.handle();
// ????μ??.
Scanner scanner = new Scanner(System.in);
while (true) {
String text = scanner.next();
// ???????????.
if ("exit".equals(text)) {
// ????????????????.
handler.sendFinish();
break;
}
handler.sendMsg(text);
}
try {
// ??????????????д????????????.
latch.await();
} catch (InterruptedException e) {
e.printStackTrace();
}
// ?????? ???????????.
handler.release();
// ???????????.
scanner.close();
}
}
??????
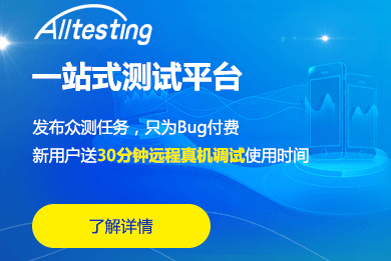
???·???
??????????????????
2023/3/23 14:23:39???д?ò??????????
2023/3/22 16:17:39????????????????????Щ??
2022/6/14 16:14:27??????????????????????????
2021/10/18 15:37:44???????????????
2021/9/17 15:19:29???·???????·
2021/9/14 15:42:25?????????????
2021/5/28 17:25:47??????APP??????????
2021/5/8 17:01:11