Net?????????CacheManager
???????????? ???????[ 2015/12/11 11:01:12 ] ???????????????????
????Cache???????????????????????????????????CPU????????棬???????棬????????л??棬???????????з???????memcache??redis????????????????????????????????????????????????????????????У?????????????????IO??????????????????????????????????????????????????????????????????????浽???????????????У??′η??????????????????????????????л?????????????????????????????????????????????.Net?????У???????CacheManager??????????????е???檔
???????CacheManager??????????
????CacheManager??????.Net????????????????????????????????????????????????????ú?????????????棬??????ó????????????????м??
??????????CacheManager???Щ???:
?????????????????????????????????棬??????????????淽????
????CacheManager????????????棬?????????appfabric??redis??couchbase??windowsazurecache??memorycache???
?????????????????綹????????????????????????????????…
????????CacheManager??????
????CacheManager???????????????????????滺????CacheManager????????????????????????????CacheManager?????á?
??????????VisualStudio?д??????ConsoleApplication.
???????Nuget???????CacheManager?????á?CacheManager?????????Package.????CacheManager.Core???????????????????????????в??????Package.
???????Demo?У?????????????????棬??????????CacheManager.Core??CacheManager.SystemRuntimeCaching
??????????Main?????????ú?????????:
????1 using System;
????2 using CacheManager.Core;
????3 namespace ConsoleApplication
????4 {
????5 class Program
????6 {
????7 static void Main(string[] args)
????8 {
????9 var cache = CacheFactory.Build("getStartedCache"?? settings =>
????10 {
????11 settings.WithSystemRuntimeCacheHandle("handleName");
????12 });
????13 }
????14 }
????15 }
?????????????????CacheFactory??????????????getStartedCache??????????????????????????SystemRunTimeCache????滺?檔?????????????????????Handle??????????????????????洢?????????????Redis????????????洢??????????????????????????????????????????????????????ú???á?
????????????????????Щ???????????
????1 static void Main(string[] args)
????2 {
????3
????4 var cache = CacheFactory.Build("getStartedCache"?? settings =>
????5 {
????6 settings.WithSystemRuntimeCacheHandle("handleName");
????7 });
????8
????9 cache.Add("keyA"?? "valueA");
????10 cache.Put("keyB"?? 23);
????11 cache.Update("keyB"?? v => 42);
????12 Console.WriteLine("KeyA is " + cache.Get("keyA")); // should be valueA
????13 Console.WriteLine("KeyB is " + cache.Get("keyB")); // should be 42
????14 cache.Remove("keyA");
????15 Console.WriteLine("KeyA removed? " + (cache.Get("keyA") == null).ToString());
????16 Console.WriteLine("We are done...");
????17 Console.ReadKey();
????18 }
????????CacheManager??????????
???????????У????????????????????檔
?????????????????????????????????????????????redis????????????????????????????????ó????????????Щ???????????????Щ?????????????????
?????????????????????????????????????????????????????????????????????????л??????紫???????????
?????????????????????????????????????????滺???????????????????????????????????ж??????????????ó?????????????
?????????滺??????????????????????100?????????????
???????CacheManager????????????????????????????
????1 var cache = CacheFactory.Build<int>("myCache"?? settings =>
????2 {
????3 settings
????4 .WithSystemRuntimeCacheHandle("inProcessCache")//??滺??Handle
????5 .And
????6 .WithRedisConfiguration("redis"?? config =>//Redis????????
????7 {
????8 config.WithAllowAdmin()
????9 .WithDatabase(0)
????10 .WithEndpoint("localhost"?? 6379);
????11 })
????12 .WithMaxRetries(1000)//???????
????13 .WithRetryTimeout(100)//?????????
????14 .WithRedisBackPlate("redis")//redis???Back Plate
????15 .WithRedisCacheHandle("redis"?? true);//redis????handle
????16 });
????????????У???滺???Redis???????ò??????????????????BackPlate????????????????????????CacheManager?е?BackPlate????????
???????BackPlate????????????е????????
???????????????????????????????????????????????????????????????????????????????????????????????????????????????????????????п?????????????μ??????
????????????A?е??????????е????????????????CacheManager????A????????е????handle?и???????????????????????????????????????????B?е???滺???У?????????????δ???μ??????????B???????????????????????????滺???????????е????????μ??????
???????????????????????????????????cachebackplate???????????????е???檔
???????????????????У??????redis???BackPlate???.???????е???????????redis?л???????????????
??????????redis???BackPlate??????????????????????μ???????????redis?е????????????????CacheManager???????????е???滺???е????????redis?е????????????
??????????????????????
?????????????????????????μ????CacheManager??????????????BackPlate?洢??ε?????仯???????????????????????????Щ?????????????????????o????????????????????????
?????壬ExpirationMode??CacheUpdateMode
?????漰?????棬????л???????????CacheManager???????Щ???????????????á?
????1 public enum ExpirationMode
????2 {
????3 None = 0??
????4 Sliding = 1??
????5 Absolute = 2??
????6 }
??????CacheManager???????????????ò??????????2???
????1 public enum CacheUpdateMode
????2 {
????3 None = 0??
????4 Full = 1??
????5 Up = 2??
????6 }
???????Sliding??Up?????????????????????ò????????????????????????????????????????????????????У????????θ?????????????С???CacheManager???????????????????????????????????????????????????Up?????????????????????浽??滺???С?
????????????÷??????:
????1 var cache = CacheFactory.Build<int>("myCache"?? settings =>
????2 {
????3 settings.WithUpdateMode(CacheUpdateMode.Up)
????4 .WithSystemRuntimeCacheHandle("inProcessCache")//??滺??Handle
????5 .WithExpiration(ExpirationMode.Sliding?? TimeSpan.FromSeconds(60)))
????6 .And
????7 .WithRedisConfiguration("redis"?? config =>//Redis????????
????8 {
????9 config.WithAllowAdmin()
????10 .WithDatabase(0)
????11 .WithEndpoint("localhost"?? 6379);
????12 }).
????13 .WithExpiration(ExpirationMode.Sliding?? TimeSpan. FromHours (24)))
????14 .WithMaxRetries(1000)//???????
????15 .WithRetryTimeout(100)//?????????
????16 .WithRedisBackPlate("redis")//redis???Back Plate
????17 .WithRedisCacheHandle("redis"?? true);//redis????handle
????18
????19 });
??????????????÷???
?????????????У????????hit??miss??????????????Щ????????????????????????????????????????????????????
????1varcache=CacheFactory.Build("cacheName"??settings=>settings
????2.WithSystemRuntimeCacheHandle("handleName")
????3.EnableStatistics()
????4.EnablePerformanceCounters());
?????????ú?????Statistic?????????????????????????????????????????????????handle?е?????????
????1 foreach (var handle in cache.CacheHandles)
????2 {
????3 var stats = handle.Stats;
????4 Console.WriteLine(string.Format(
????5 "Items: {0}?? Hits: {1}?? Miss: {2}?? Remove: {3}?? ClearRegion: {4}?? Clear: {5}?? Adds: {6}?? Puts: {7}?? Gets: {8}"??
????6 stats.GetStatistic(CacheStatsCounterType.Items)??
????7 stats.GetStatistic(CacheStatsCounterType.Hits)??
????8 stats.GetStatistic(CacheStatsCounterType.Misses)??
????9 stats.GetStatistic(CacheStatsCounterType.RemoveCalls)??
????10 stats.GetStatistic(CacheStatsCounterType.ClearRegionCalls)??
????11 stats.GetStatistic(CacheStatsCounterType.ClearCalls)??
????12 stats.GetStatistic(CacheStatsCounterType.AddCalls)??
????13 stats.GetStatistic(CacheStatsCounterType.PutCalls)??
????14 stats.GetStatistic(CacheStatsCounterType.GetCalls)
????15 ));
????16 }
?????????β
???????????????????ú????????????????????????????????????????????????????????????????????CachManager????á?
??????
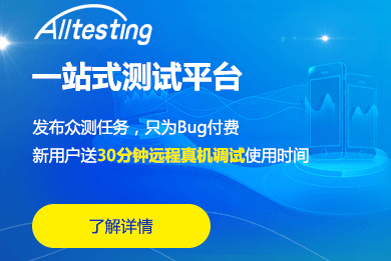
???·???
??????????????????
2023/3/23 14:23:39???д?ò??????????
2023/3/22 16:17:39????????????????????Щ??
2022/6/14 16:14:27??????????????????????????
2021/10/18 15:37:44???????????????
2021/9/17 15:19:29???·???????·
2021/9/14 15:42:25?????????????
2021/5/28 17:25:47??????APP??????????
2021/5/8 17:01:11