C++??????????????
???????????? ???????[ 2015/11/25 13:32:48 ] ??????????????????? .NET
????Stack.hpp
#pragma once
template <class T>
class Stack{
private:
T* _array;
size_t _capacity;
int _topindex;
public:
Stack() //????????
:_array(0)
?? _capacity(0)
?? _topindex(-1)
{}
void Push(const T& x){ //???????
if (_topindex + 1 == _capacity){
_capacity = 2 * _capacity + 3;
T* tmp = new T[_capacity];
if (tmp == NULL){
cout << "failed new" << endl;
exit(-1);
}
memcpy(tmp?? _array?? sizeof(T) * (_topindex + 1));
delete _array;
_array = tmp;
}
_array[++_topindex] = x;
}
void Pop(){ //???
if (_topindex > -1){
//cout << _array[_topindex] << endl;
_topindex--;
}
}
bool empty(){ //???
return _topindex = -1;
}
const T& top(){ //???????
//cout << _array[_topindex] << endl;
return _array[_topindex];
}
};
main.cpp
#include<iostream>
#include<string>
#include"Stack.hpp"
using namespace std;
enum Type{
ADD??
SUB??
MUL??
DIV??
OP_NUM??
};
struct Cell{
Type _type;
int num;
};
Cell RPNExp[] = {
OP_NUM?? 12??
OP_NUM?? 3??
OP_NUM?? 4??
ADD?? 1??
MUL?? 1??
OP_NUM?? 6??
SUB?? 1??
OP_NUM?? 8??
OP_NUM?? 2??
DIV?? 1??
ADD?? 1??
};
int main(){
Stack<int> s2;
int i = 0;
for (int i = 0; i < sizeof(RPNExp) / sizeof(RPNExp[0]);i++){ //??????
int left?? right; //??????????
switch (RPNExp[i]._type){ //???????????
case ADD: //???????????????????
left = s2.top();
s2.Pop();
right = s2.top();
s2.Pop();
s2.Push(left + right); //?μ?????
break;
case SUB: //????????????????????
left = s2.top();
s2.Pop();
right = s2.top();
s2.Pop();
s2.Push(right - left);
break;
case MUL: //???????????????????
left = s2.top();
s2.Pop();
right = s2.top();
s2.Pop();
s2.Push(left * right);
break;
case DIV: //????????????????????
left = s2.top();
s2.Pop();
right = s2.top();
s2.Pop();
s2.Push(right / left);
break;
case OP_NUM: //???????????
s2.Push(RPNExp[i].num);
break;
default:
return -1;
}
}
cout << s2.top() << endl;
return 0;
}
??????
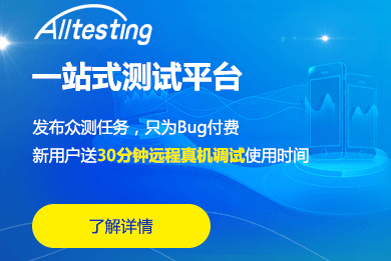
???·???
??????????????????
2023/3/23 14:23:39???д?ò??????????
2023/3/22 16:17:39????????????????????Щ??
2022/6/14 16:14:27??????????????????????????
2021/10/18 15:37:44???????????????
2021/9/17 15:19:29???·???????·
2021/9/14 15:42:25?????????????
2021/5/28 17:25:47??????APP??????????
2021/5/8 17:01:11