C#???jQuery????????
???????????? ???????[ 2015/11/12 10:52:59 ] ???????????????????
????jQuery????????????????????????????????????????????????C#????????????????????????????????????????о??????jQuery????????????????????????????????????????????ɡ????javascript?????C#???????????
??????????????д???jQPerson??????÷??????????ID??Name??Age????????????????????????
1 using System;
2 using System.Collections.Generic;
3 using System.Linq;
4 using System.Text;
5 using System.Threading.Tasks;
6
7 namespace CSharpMethodLikeJQuery
8 {
9 public class jQPerson
10 {
11 string Id { set; get; }
12 string Name { set; get; }
13 int Age { set; get; }
14 string Sex { set; get; }
15 string Info { set; get; }
16
17 public jQPerson()
18 {
19
20 }
21 /// <summary>
22 /// ????ID??????this????jQPerson???
23 /// </summary>
24 /// <param name="Id"></param>
25 /// <returns></returns>
26 public jQPerson setId(string Id)
27 {
28 this.Id = Id;
29 return this;
30 }
31 /// <summary>
32 /// ????this????jQPerson???
33 /// </summary>
34 /// <param name="name"></param>
35 /// <returns></returns>
36 public jQPerson setName(string name)
37 {
38
39 this.Name = name;
40 return this;
41 }
42 /// <summary>
43 /// ????this????jQPerson???
44 /// </summary>
45 /// <param name="age"></param>
46 /// <returns></returns>
47 public jQPerson setAge(int age)
48 {
49
50 this.Age = age;
51 return this;
52 }
53 /// <summary>
54 /// ????this????jQPerson???
55 /// </summary>
56 /// <param name="sex"></param>
57 /// <returns></returns>
58 public jQPerson setSex(string sex)
59 {
60
61 this.Sex = sex;
62 return this;
63 }
64 /// <summary>
65 /// ????this????jQPerson???
66 /// </summary>
67 /// <param name="info"></param>
68 /// <returns></returns>
69 public jQPerson setInfo(string info)
70 {
71
72 this.Info = info;
73 return this;
74 }
75 /// <summary>
76 /// tostring???????????
77 /// </summary>
78 /// <returns></returns>
79 public string toString()
80 {
81
82 return string.Format("Id:{0}??Name:{1}??Age:{2}??Sex:{3}??Info:{4}"?? this.Id?? this.Name?? this.Age?? this.Sex?? this.Info);
83
84
85 }
86
87 }
88 }
?????????????????в??????????????????Ч??
1 /// <summary>
2 ///toString ?????
3 ///</summary>
4 [TestMethod()]
5 public void toStringTest()
6 {
7 jQPerson target = new jQPerson();
8 target.setId("2")
9 .setName("jack")
10 .setAge(26)
11 .setSex("man")
12 .setInfo("ok");
13 string expected = "Id:2??Name:jack??Age:26??Sex:man??Info:ok";
14 string actual;
15 actual = target.toString();
16 Assert.AreEqual(expected?? actual);
17 //Assert.Inconclusive("???????????????????");
18 }
??????????????????????????????????????????????????
??????
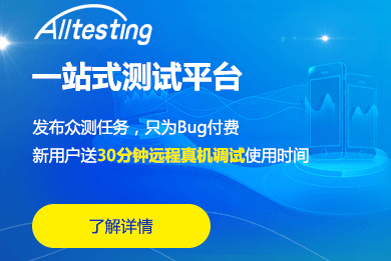
???·???
??????????????????
2023/3/23 14:23:39???д?ò??????????
2023/3/22 16:17:39????????????????????Щ??
2022/6/14 16:14:27??????????????????????????
2021/10/18 15:37:44???????????????
2021/9/17 15:19:29???·???????·
2021/9/14 15:42:25?????????????
2021/5/28 17:25:47??????APP??????????
2021/5/8 17:01:11